试设计一个算术四则运算表达式求值的简单计算器。 基本要求: 1、 操作数均为非负整数常数,操作符仅为+、-、*、/、、; 2、 编写 main 函数进行测试
时间: 2023-10-20 10:09:16 浏览: 82
好的,我可以给你设计一个简单的计算器代码示例:
```
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool isDigit(char c) {
return c >= '0' && c <= '9';
}
int calc(int num1, int num2, char op) {
if (op == '+') return num1 + num2;
if (op == '-') return num1 - num2;
if (op == '*') return num1 * num2;
if (op == '/') return num1 / num2;
if (op == '%') return num1 % num2;
}
int eval(string expr) {
stack<int> nums;
stack<char> ops;
int num = 0;
bool hasNum = false;
for (int i = 0; i < expr.length(); i++) {
char c = expr[i];
if (isDigit(c)) {
num = num * 10 + (c - '0');
hasNum = true;
} else if (hasNum) {
nums.push(num);
num = 0;
hasNum = false;
}
if (c == '(') {
ops.push(c);
} else if (c == ')') {
while (ops.top() != '(') {
int num2 = nums.top(); nums.pop();
int num1 = nums.top(); nums.pop();
char op = ops.top(); ops.pop();
nums.push(calc(num1, num2, op));
}
ops.pop();
} else if (c == '+' || c == '-') {
while (!ops.empty() && ops.top() != '(') {
int num2 = nums.top(); nums.pop();
int num1 = nums.top(); nums.pop();
char op = ops.top(); ops.pop();
nums.push(calc(num1, num2, op));
}
ops.push(c);
} else if (c == '*' || c == '/' || c == '%') {
while (!ops.empty() && ops.top() != '(' &&
(c == '*' || c == '/' || ops.top() == '%')) {
int num2 = nums.top(); nums.pop();
int num1 = nums.top(); nums.pop();
char op = ops.top(); ops.pop();
nums.push(calc(num1, num2, op));
}
ops.push(c);
}
}
if (hasNum) {
nums.push(num);
}
while (!ops.empty()) {
int num2 = nums.top(); nums.pop();
int num1 = nums.top(); nums.pop();
char op = ops.top(); ops.pop();
nums.push(calc(num1, num2, op));
}
return nums.top();
}
int main() {
string expr = "3+4*2";
cout << "Input expression: " << expr << endl;
int result = eval(expr);
cout << "Result: " << result << endl;
return 0;
}
```
这个计算器支持加、减、乘、除、取余五种运算符,并且可以处理表达式中的括号。你可以将expr的值更改为其他算术表达式进行测试。
相关推荐

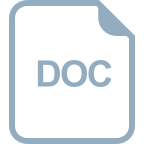
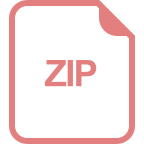
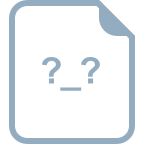
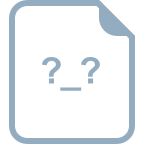
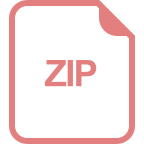
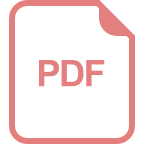