JAVA线程实时读取另一个线程的数据
时间: 2023-09-23 20:12:07 浏览: 49
可以使用管道(PipedInputStream和PipedOutputStream)来实现一个线程读取另一个线程的数据。具体步骤如下:
1. 在一个线程中创建PipedInputStream和PipedOutputStream对象,并将它们连接起来。
2. 在另一个线程中,将需要实时读取的数据写入PipedOutputStream对象中。
3. 在第一个线程中,通过PipedInputStream对象读取数据。
示例代码如下:
```
import java.io.IOException;
import java.io.PipedInputStream;
import java.io.PipedOutputStream;
public class Main {
public static void main(String[] args) throws IOException {
PipedInputStream in = new PipedInputStream();
PipedOutputStream out = new PipedOutputStream(in);
Thread writerThread = new Thread(() -> {
try {
out.write("Hello world".getBytes());
} catch (IOException e) {
e.printStackTrace();
}
});
Thread readerThread = new Thread(() -> {
try {
int data;
while ((data = in.read()) != -1) {
System.out.print((char) data);
}
} catch (IOException e) {
e.printStackTrace();
}
});
writerThread.start();
readerThread.start();
}
}
```
在上面的示例中,writerThread线程将字符串"Hello world"写入PipedOutputStream对象中,而readerThread线程从PipedInputStream对象中读取数据并输出到控制台上。
相关推荐
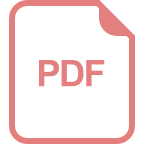














