用C语言编写一个程序,读入一行字符,且每个字符存入一个结点,按输入顺序建立一个链表的结点序列,然后再按相反顺序输出并释放全部结点。 函数接口定义: 在这里描述函数接口。例如: struct Node *create(); void print(struct Node *head); 函数create,要求从表尾开始逆向建立链表;函数print,要求相反顺序输出并释放全部结点。 裁判测试程序样例: #include <stdio.h> #include <malloc.h> struct Node { char info; struct Node *link; }; struct Node *create(); void print(struct Node *head); int main() { struct Node head; head=create(); print(head); return 0; } / 你的代码将被嵌在这里 */
时间: 2024-02-03 13:04:31 浏览: 37
在主函数中创建结构体头节点是错误的做法,应该改为创建一个指针类型的头节点,并将头节点的指针传递给create函数。另外,在print函数中需要使用一个中间变量来保存当前节点的指针,以避免释放节点后无法访问下一个节点的问题。以下是修改后的代码:
```
#include <stdio.h>
#include <stdlib.h>
struct Node {
char info;
struct Node *link;
};
struct Node *create();
void print(struct Node *head);
int main() {
struct Node *head;
head = create();
print(head);
return 0;
}
struct Node *create() {
struct Node *head = NULL;
struct Node *temp = NULL;
char c;
c = getchar();
while (c != '\n') {
temp = (struct Node*)malloc(sizeof(struct Node));
temp->info = c;
temp->link = head;
head = temp;
c = getchar();
}
return head;
}
void print(struct Node *head) {
struct Node *temp;
while (head != NULL) {
printf("%c", head->info);
temp = head;
head = head->link;
free(temp);
}
}
```
相关推荐
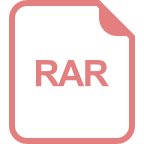















