设计字符串类CSTRING,包含私有成员数据p(char *p)。根据给定的main函数设计必要的成员函数
时间: 2024-02-15 19:04:55 浏览: 35
根据给定的 `main` 函数,我们可以得知 `CSTRING` 需要包含以下成员函数:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class CSTRING {
private:
char *p;
public:
CSTRING(const char *str = nullptr); // 构造函数
CSTRING(const CSTRING &str); // 拷贝构造函数
~CSTRING(); // 析构函数
void print() const; // 输出字符串
void append(const char *str); // 追加字符串
};
CSTRING::CSTRING(const char *str) {
if (str == nullptr) {
p = new char[1];
*p = '\0';
} else {
p = new char[strlen(str) + 1];
strcpy(p, str);
}
}
CSTRING::CSTRING(const CSTRING &str) {
p = new char[strlen(str.p) + 1];
strcpy(p, str.p);
}
CSTRING::~CSTRING() {
delete[] p;
}
void CSTRING::print() const {
cout << p << endl;
}
void CSTRING::append(const char *str) {
char *tmp = new char[strlen(p) + strlen(str) + 1];
strcpy(tmp, p);
strcat(tmp, str);
delete[] p;
p = tmp;
}
int main() {
CSTRING str1("Hello");
CSTRING str2(str1);
str1.print();
str2.print();
str1.append(" World");
str1.print();
str2.print();
return 0;
}
```
在上述代码中,`CSTRING` 类的成员函数包括:
- 构造函数:用于初始化字符串,如果传入的字符串为空指针,则将 `p` 指向一个空字符;否则,根据传入的字符串长度动态分配内存,并将值复制到 `p` 中。
- 拷贝构造函数:用于实现深拷贝,即动态分配内存并将值复制到新的 `CSTRING` 对象中。
- 析构函数:用于释放 `p` 指向的内存。
- `print` 函数:用于输出 `p` 指向的字符串。
- `append` 函数:用于将传入的字符串追加到 `p` 指向的字符串末尾。需要先动态分配一个新的字符数组 `tmp`,将 `p` 的值复制到 `tmp` 中,再将追加的字符串复制到 `tmp` 末尾,最后释放 `p` 指向的内存,将 `p` 指向 `tmp`。
这样,我们就完成了 `CSTRING` 类的设计。
相关推荐
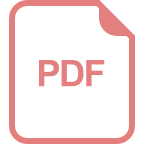
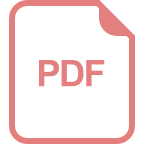








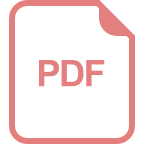
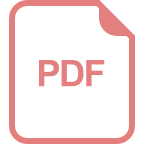
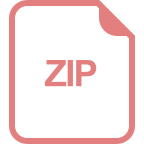