设计字符串类CSTRING,包含私有成员数据p(char *p)。根据给定的main函数设计必要的成员函数。
时间: 2024-02-18 12:05:58 浏览: 82
以下是CSTRING类的设计和必要的成员函数实现:
```cpp
class CSTRING {
private:
char *p;
public:
// 默认构造函数
CSTRING() {
p = nullptr;
}
// 带参构造函数
CSTRING(const char *str) {
if (str != nullptr) {
int len = strlen(str);
p = new char[len + 1];
strcpy(p, str);
} else {
p = nullptr;
}
}
// 拷贝构造函数
CSTRING(const CSTRING &cstr) {
if (cstr.p != nullptr) {
int len = strlen(cstr.p);
p = new char[len + 1];
strcpy(p, cstr.p);
} else {
p = nullptr;
}
}
// 析构函数
~CSTRING() {
if (p != nullptr) {
delete[] p;
p = nullptr;
}
}
// 赋值运算符重载
CSTRING &operator=(const CSTRING &cstr) {
if (this == &cstr) {
return *this;
}
if (cstr.p != nullptr) {
int len = strlen(cstr.p);
if (p != nullptr) {
delete[] p;
}
p = new char[len + 1];
strcpy(p, cstr.p);
} else {
if (p != nullptr) {
delete[] p;
}
p = nullptr;
}
return *this;
}
// 字符串长度
int length() const {
if (p != nullptr) {
return strlen(p);
} else {
return 0;
}
}
// 字符串拼接
CSTRING operator+(const CSTRING &cstr) const {
if (cstr.p == nullptr) {
return *this;
}
if (p == nullptr) {
return cstr;
}
CSTRING result;
int len1 = strlen(p);
int len2 = strlen(cstr.p);
result.p = new char[len1 + len2 + 1];
strcpy(result.p, p);
strcat(result.p, cstr.p);
return result;
}
// 字符串比较
bool operator==(const CSTRING &cstr) const {
if (p == nullptr && cstr.p == nullptr) {
return true;
}
if (p == nullptr || cstr.p == nullptr) {
return false;
}
return strcmp(p, cstr.p) == 0;
}
// 字符串索引访问
char &operator[](int index) const {
if (index < 0 || index >= length()) {
throw "Index out of range.";
}
return p[index];
}
// 字符串输出
friend ostream &operator<<(ostream &out, const CSTRING &cstr) {
if (cstr.p != nullptr) {
out << cstr.p;
}
return out;
}
};
```
以下是一个使用CSTRING类的示例main函数:
```cpp
int main() {
CSTRING str1("Hello");
CSTRING str2("World");
CSTRING str3 = str1 + str2;
cout << "str1: " << str1 << endl;
cout << "str2: " << str2 << endl;
cout << "str3: " << str3 << endl;
if (str1 == str2) {
cout << "str1 equals str2" << endl;
} else {
cout << "str1 does not equal str2" << endl;
}
try {
cout << "str1[10]: " << str1[10] << endl;
} catch (const char *msg) {
cerr << "Error: " << msg << endl;
}
return 0;
}
```
阅读全文
相关推荐
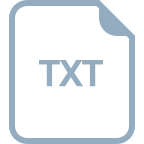







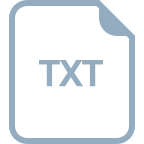
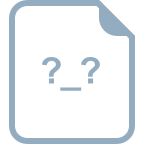
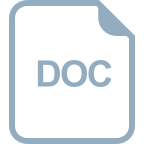

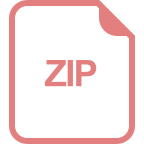
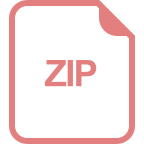