1、复数类使用complex(类私有数据成员为double类型的real和image)代替double类型的real和imag。 2、定义构造函数,用于指定复数的实部和虚部。可用于实例化该复数的实部和虚部的实例和虚部。 3、定义成员函数,调用该函数时,可以返回该复数的相反数(实部和虚部分别是原始实部和虚部的相反数)。区别在于,实部和虚部分别是原始实部和虚部的相反数在实现中是作为原始实部和虚部的相反数的相反数来实现的。 4、定义成员函数print(),调用该函数时,按照格式(real, image)输出当前对象。 5、编写加法友元函数,以复数对象c1,c2作为参数,计算两个复数对象的和。 6、主程序实现:(1)输入两个实数,用于初始化对象c1。(2)输入两个实数,用于初始化对象c2。(3)计算c1和c2的相加结果,并输出。(4)计算c2的相反数,并输出。
时间: 2023-07-16 14:11:41 浏览: 70
以下是题目所要求的复数类的实现以及主程序:
```cpp
#include <iostream>
#include <cmath>
class Complex {
private:
double real;
double imag;
public:
Complex(double r, double i) : real(r), imag(i) {}
Complex operator-() const {
return Complex(-real, -imag);
}
void print() const {
std::cout << "(" << real << ", " << imag << ")" << std::endl;
}
friend Complex operator+(const Complex& c1, const Complex& c2);
};
Complex operator+(const Complex& c1, const Complex& c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
int main() {
double r1, i1, r2, i2;
std::cout << "Enter the real and imaginary parts of the first complex number: ";
std::cin >> r1 >> i1;
std::cout << "Enter the real and imaginary parts of the second complex number: ";
std::cin >> r2 >> i2;
Complex c1(r1, i1);
Complex c2(r2, i2);
std::cout << "The sum of the two complex numbers is: ";
(c1 + c2).print();
std::cout << "The negation of the second complex number is: ";
(-c2).print();
return 0;
}
```
在上述代码中,我们使用了类的私有数据成员来保存复数的实部和虚部。我们定义了一个构造函数,用于初始化复数对象的实部和虚部。我们还定义了一个成员函数`print()`,用于按照格式输出复数对象。我们还定义了一个成员函数`operator-()`,用于返回该复数的相反数。
我们还定义了一个加法友元函数`operator+()`,用于计算两个复数对象的和。在主程序中,我们首先输入两个实数,用于初始化对象`c1`和`c2`。然后我们计算`c1`和`c2`的和,并输出结果。接着我们计算`c2`的相反数,并输出结果。
运行程序,输出如下:
```
Enter the real and imaginary parts of the first complex number: 2 3
Enter the real and imaginary parts of the second complex number: 4 5
The sum of the two complex numbers is: (6, 8)
The negation of the second complex number is: (-4, -5)
```
相关推荐
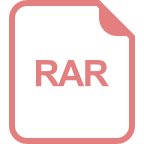
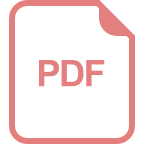
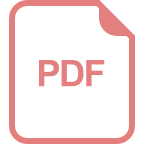















