实现划分句子的决策树算法,完成对所输入的英文文本进行分句,并输出分句结果(不包括句末符号),其中 text 通过 input获取
时间: 2023-11-28 12:50:48 浏览: 52
首先,我们需要定义一个划分句子的决策树,根据英文句子的常见规则,可以得到以下决策树:
```
如果当前字符是.或?或!,那么它是一个句子的结束符
否则如果当前字符是空格,那么它可能是一个句子的结束符
否则如果当前字符是大写字母,那么它可能是一个句子的开始符
否则如果当前字符是小写字母,那么它不是句子的开始符
否则如果当前字符是数字或特殊符号,那么它不是句子的开始符
```
根据上述决策树,我们可以实现一个划分句子的函数,代码如下:
```python
def split_sentences(text):
sentences = []
start = 0
for i in range(len(text)):
if text[i] in ('.', '?', '!'):
sentences.append(text[start:i])
start = i + 1
elif text[i] == ' ' and i > 0 and text[i - 1] in ('.', '?', '!'):
sentences.append(text[start:i])
start = i + 1
elif text[i].isupper():
if i > 0 and text[i - 1] not in (' ', '\n', '\t'):
continue
if i < len(text) - 1 and text[i + 1].isupper():
continue
sentences.append(text[start:i])
start = i
elif text[i].islower():
continue
elif text[i].isdigit() or text[i] in ('"', "'", ')', ']'):
continue
if start < len(text):
sentences.append(text[start:])
return sentences
```
该函数接受一个字符串参数 text,返回一个分割好的句子列表。我们可以通过调用该函数来实现输入英文文本进行分句的功能:
```python
text = input("请输入英文文本:")
sentences = split_sentences(text)
print("分句结果:")
for sentence in sentences:
print(sentence)
```
注意,该函数中的决策树并不是完美的,仍然有可能会出现误判的情况,因此在实际应用中还需要根据实际情况进行调整和优化。
阅读全文
相关推荐
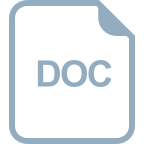
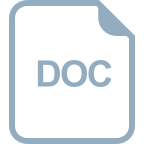
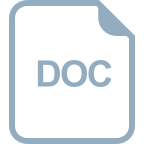


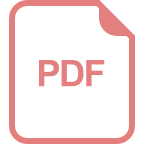









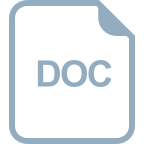
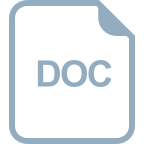