用MATLAB代码实现道格拉斯普克算法的去阈值化
时间: 2024-10-27 16:08:59 浏览: 41
在MATLAB中,你可以使用道格拉斯-佩克算法(Douglas-Peucker Algorithm)来简化复杂的二维曲线。这个算法主要用于减少曲线上的点数,保留最重要的特征。下面是一个简单的实现示例:
```matlab
function [new_points, distances] = dp_algorithm(points, epsilon)
if length(points) <= 2
return points, zeros(1, length(points)); % 如果只有两个点,返回原点
end
% 第一个和最后一个点总是保留
new_points = [points(1), points(end)];
% 初始化距离列表
distances = zeros(1, length(points));
% 计算起点到终点之间的距离
dist_start_end = norm(points(2,:) - points(1,:));
% 遍历剩余点
for i = 2:length(points) - 1
% 计算当前点到已选点的最短距离
current_dist = distance_between(new_points, points(i,:), 'euclidean');
% 如果当前点到起点的距离加上最近点到起点的距离大于给定的epsilon,则添加点
if dist_start_end + min(distances(1:i-1)) > epsilon
new_points = [new_points, points(i,:)];
distances(i) = current_dist;
else
distances(i) = Inf; % 无限大,表示不加入该点
end
end
% 返回新点和对应的距离
end
function d = distance_between(point1, point2, metric)
switch metric
case 'euclidean'
d = norm(point1 - point2);
otherwise
error('Unsupported metric');
end
end
% 使用示例
points = ...; % 你的二维坐标数据
epsilon = 0.1; % 阈值,可以根据需要调整
[new_points, distances] = dp_algorithm(points, epsilon);
plot(points(:,1), points(:,2), '.b'); % 原始曲线
hold on;
plot(new_points(:,1), new_points(:,2), 'ro'); % 去阈值化后的曲线
hold off;
```
在这个例子中,你需要提供一个二维坐标数据`points`和一个阈值`epsilon`。`distance_between`函数用于计算两点之间的欧式距离。
阅读全文
相关推荐
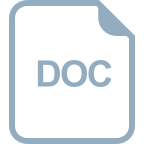
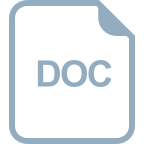
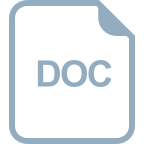
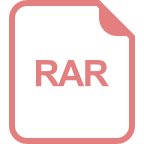
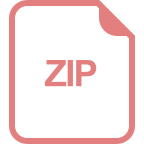
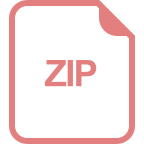
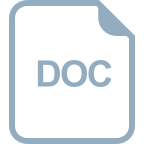
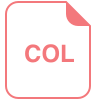

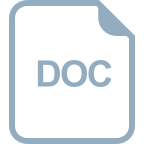
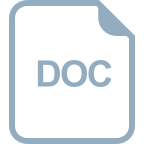
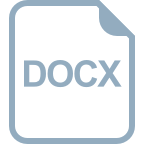
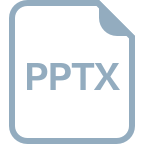
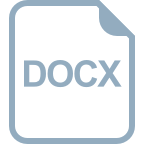
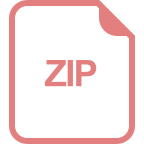
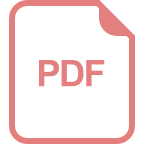
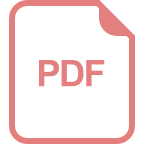
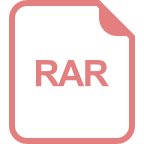
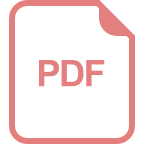