对任意输入的一组数据,建立一个递增有序的单链表
时间: 2023-05-12 08:03:46 浏览: 33
可以使用插入排序来建立一个递增有序的单链表。具体步骤如下:
1. 创建一个空链表。
2. 依次将输入的数据插入到链表中,插入时需要保证链表的有序性。
3. 返回建立好的链表。
以下是示例代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
ListNode* insert(ListNode *head, int val) {
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
node->val = val;
node->next = NULL;
if (head == NULL) {
head = node;
} else {
ListNode *cur = head;
ListNode *prev = NULL;
while (cur != NULL && cur->val < val) {
prev = cur;
cur = cur->next;
}
if (prev == NULL) {
node->next = head;
head = node;
} else {
prev->next = node;
node->next = cur;
}
}
return head;
}
ListNode* createList(int *nums, int size) {
ListNode *head = NULL;
for (int i = 0; i < size; i++) {
head = insert(head, nums[i]);
}
return head;
}
void printList(ListNode *head) {
ListNode *cur = head;
while (cur != NULL) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
}
int main() {
int nums[] = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5};
int size = sizeof(nums) / sizeof(int);
ListNode *head = createList(nums, size);
printList(head);
return 0;
}
```
对于输入的数据 {3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5},程序输出的结果为:1 1 2 3 3 4 5 5 5 6 9。
相关推荐







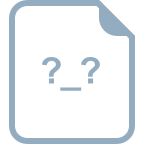
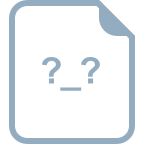
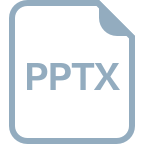