用cpp完成以下代码:对任意输入的一组数据,建立一个递增有序的单链表。 将单链表拆分成两个单链表,其中一个全为奇数,另一个全为偶数(尽量利用原存储空间)。
时间: 2023-05-17 17:05:32 浏览: 141
以下是用 C++ 完成的代码:
```cpp
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* createList() {
ListNode* head = new ListNode(0);
ListNode* p = head;
int x;
while (cin >> x) {
ListNode* node = new ListNode(x);
p->next = node;
p = node;
}
return head->next;
}
void splitList(ListNode* head, ListNode*& oddHead, ListNode*& evenHead) {
ListNode* odd = new ListNode(0);
ListNode* even = new ListNode(0);
ListNode* p = head;
ListNode* oddP = odd;
ListNode* evenP = even;
while (p) {
if (p->val % 2 == 0) {
evenP->next = p;
evenP = p;
} else {
oddP->next = p;
oddP = p;
}
p = p->next;
}
evenP->next = NULL;
oddP->next = NULL;
evenHead = even->next;
oddHead = odd->next;
}
void printList(ListNode* head) {
ListNode* p = head;
while (p) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
}
int main() {
ListNode* head = createList();
ListNode* oddHead, * evenHead;
splitList(head, oddHead, evenHead);
cout << "Odd List: ";
printList(oddHead);
cout << "Even List: ";
printList(evenHead);
return 0;
}
```
这个程序会从标准输入读入一组数据,然后建立一个递增有序的单链表。接着,它会将单链表拆分成两个单链表,其中一个全为奇数,另一个全为偶数,并尽量利用原存储空间。最后,它会输出拆分后的两个单链表。
阅读全文
相关推荐
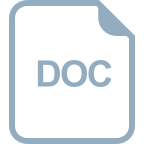
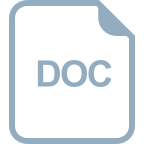
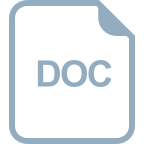














