通过数据结构的知识编写一个程序。建立单链表,在单链表中实现插入,删除和查找操作。将两个递增的有序单链表合并成一个非递减的有序单链表,不能用Python编程
时间: 2024-09-30 18:12:59 浏览: 40
在没有Python的情况下,我们可以选择一种常见的编程语言如C++来实现这个功能。首先,我们定义一个链表节点的数据结构:
```cpp
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
```
接下来,我们实现单链表的基本操作:
1. 插入元素:
```cpp
void insert(ListNode*& head, int val) {
ListNode* newNode = new ListNode(val);
if (!head) {
head = newNode;
} else {
ListNode* curr = head;
while (curr->next && curr->next->val < val) {
curr = curr->next;
}
newNode->next = curr->next;
curr->next = newNode;
}
}
```
2. 删除元素:
```cpp
ListNode* deleteNode(ListNode* head, int target) {
if (!head || !head->val) return head;
if (head->val == target) {
ListNode* temp = head;
head = head->next;
delete temp;
return head;
}
ListNode* curr = head;
while (curr->next && curr->next->val != target) {
curr = curr->next;
}
if (curr->next) {
curr->next = curr->next->next;
delete curr->next;
}
return head;
}
```
3. 查找元素:
```cpp
bool search(ListNode* head, int target) {
ListNode* curr = head;
while (curr) {
if (curr->val == target) return true;
curr = curr->next;
}
return false;
}
```
然后,合并两个递增有序链表:
```cpp
ListNode* mergeSortedLists(ListNode* l1, ListNode* l2) {
ListNode* dummyHead = new ListNode(0);
ListNode* tail = dummyHead;
while (l1 && l2) {
if (l1->val <= l2->val) {
tail->next = l1;
l1 = l1->next;
} else {
tail->next = l2;
l2 = l2->next;
}
tail = tail->next;
}
if (l1) tail->next = l1;
else tail->next = l2;
return dummyHead->next;
}
```
阅读全文
相关推荐
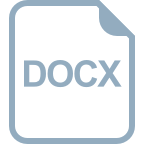
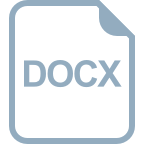


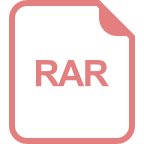
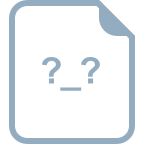
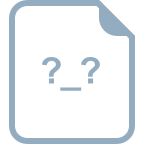
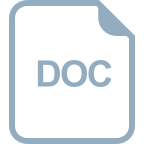
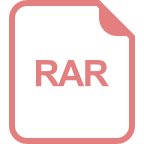
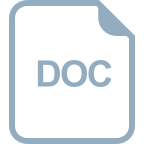
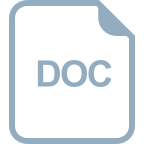
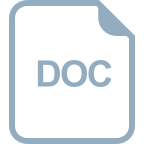
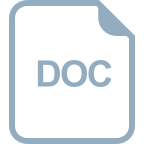
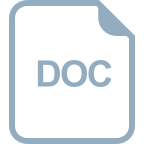
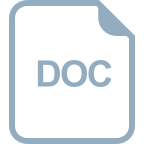
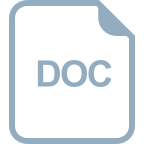