将两个递增有序的单链表的合并为一个递减有序的单链表并返回头指针C语言代码
时间: 2024-09-28 16:17:11 浏览: 54
在C语言中,合并两个递增有序的单链表并使其变为递减有序,可以采用迭代的方式。首先创建一个新的链表,然后遍历两个输入链表,每次将当前节点值较小的那个添加到新链表,并更新相应的指针。以下是代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode dummy = {0, NULL}, *head = &dummy;
while (l1 && l2) {
if (l1->val > l2->val) {
head->next = l1;
l1 = l1->next;
} else {
head->next = l2;
l2 = l2->next;
}
head = head->next;
}
if (l1)
head->next = l1;
else
head->next = l2;
return dummy.next; // 返回新链表的头节点
}
// 创建链表节点函数
ListNode* createNode(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->val = value;
newNode->next = NULL;
return newNode;
}
// 打印链表函数
void printList(ListNode* node) {
while (node) {
printf("%d ", node->val);
node = node->next;
}
printf("\n");
}
int main() {
// 测试例子
ListNode* l1 = createNode(5);
l1->next = createNode(4);
l1->next->next = createNode(3);
ListNode* l2 = createNode(6);
l2->next = createNode(2);
l2->next->next = createNode(1);
ListNode* result = mergeTwoLists(l1, l2);
printList(result); // 输出:3 2 1 4 5 6
return 0;
}
```
阅读全文
相关推荐
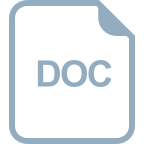
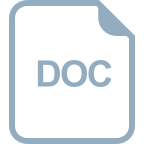
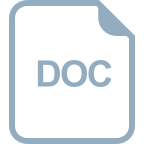













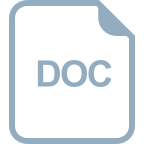
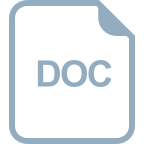
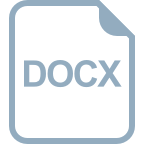