将两个带头节点的递增有序的单链表A和B合并到一个单链表C中,单链表C仍然递增有序。C语言代码
时间: 2023-08-31 14:23:13 浏览: 316
### 回答1:
以下是将两个带头节点的递增有序的单链表A和B合并到一个单链表C中,单链表C仍然递增有序的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* merge(Node* headA, Node* headB) {
Node* headC = (Node*)malloc(sizeof(Node));
Node* tailC = headC;
Node* p = headA->next;
Node* q = headB->next;
while (p && q) {
if (p->data <= q->data) {
tailC->next = p;
p = p->next;
} else {
tailC->next = q;
q = q->next;
}
tailC = tailC->next;
}
if (p) {
tailC->next = p;
} else {
tailC->next = q;
}
return headC;
}
int main() {
Node* headA = (Node*)malloc(sizeof(Node));
Node* headB = (Node*)malloc(sizeof(Node));
Node* node1 = (Node*)malloc(sizeof(Node));
node1->data = 1;
headA->next = node1;
Node* node2 = (Node*)malloc(sizeof(Node));
node2->data = 3;
node1->next = node2;
Node* node3 = (Node*)malloc(sizeof(Node));
node3->data = 5;
node2->next = node3;
node3->next = NULL;
Node* node4 = (Node*)malloc(sizeof(Node));
node4->data = 2;
headB->next = node4;
Node* node5 = (Node*)malloc(sizeof(Node));
node5->data = 4;
node4->next = node5;
Node* node6 = (Node*)malloc(sizeof(Node));
node6->data = 6;
node5->next = node6;
node6->next = NULL;
Node* headC = merge(headA, headB);
Node* p = headC->next;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return ;
}
```
### 回答2:
将两个带头节点的递增有序的单链表A和B合并到一个单链表C中,单链表C仍然递增有序的C代码如下:
```c
#include<stdio.h>
#include<stdlib.h>
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
ListNode* createNode(int val) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->val = val;
newNode->next = NULL;
return newNode;
}
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode dummy;
ListNode* tail = &dummy;
while (l1 && l2) {
if (l1->val < l2->val) {
tail->next = l1;
l1 = l1->next;
} else {
tail->next = l2;
l2 = l2->next;
}
tail = tail->next;
}
tail->next = l1 ? l1 : l2;
return dummy.next;
}
int main() {
ListNode* l1 = createNode(2);
l1->next = createNode(4);
l1->next->next = createNode(6);
ListNode* l2 = createNode(1);
l2->next = createNode(3);
l2->next->next = createNode(5);
ListNode* merged = mergeTwoLists(l1, l2);
ListNode* curr = merged;
while (curr) {
printf("%d ", curr->val);
curr = curr->next;
}
return 0;
}
```
运行以上代码,将会输出合并后的单链表C的元素:1 2 3 4 5 6。
### 回答3:
实现这个问题可以使用两个指针分别指向两个链表的头节点,比较两个节点的值,较小的节点插入到链表C中,同时更新对应的指针指向下一个节点,直到其中一个链表遍历完毕,然后将剩余的链表直接连接到链表C的尾部。
以下是C语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* merge(struct ListNode* headA, struct ListNode* headB) {
struct ListNode dummy; // 设定一个哑节点dummy作为链表C的头节点
struct ListNode* cur = &dummy; // cur指向链表C的当前节点
// 当两个链表都不为空时,比较节点的值,较小的节点插入到链表C中
while (headA && headB) {
if (headA->val <= headB->val) {
cur->next = headA;
headA = headA->next;
} else {
cur->next = headB;
headB = headB->next;
}
cur = cur->next;
}
// 将剩余的链表连接到链表C的尾部
if (headA) {
cur->next = headA;
} else {
cur->next = headB;
}
return dummy.next;
}
// 创建一个单链表
struct ListNode* createList(int arr[], int n) {
struct ListNode dummy;
struct ListNode* cur = &dummy;
for (int i = 0; i < n; i++) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = arr[i];
newNode->next = NULL;
cur->next = newNode;
cur = cur->next;
}
return dummy.next;
}
// 打印单链表
void printList(struct ListNode* head) {
struct ListNode* cur = head;
while (cur) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
}
int main() {
int arrA[] = {1, 3, 5};
int arrB[] = {2, 4, 6};
int lenA = sizeof(arrA) / sizeof(arrA[0]);
int lenB = sizeof(arrB) / sizeof(arrB[0]);
struct ListNode* headA = createList(arrA, lenA);
struct ListNode* headB = createList(arrB, lenB);
printf("链表A:");
printList(headA);
printf("链表B:");
printList(headB);
struct ListNode* headC = merge(headA, headB);
printf("合并后链表C:");
printList(headC);
return 0;
}
```
运行结果:
```
链表A:1 3 5
链表B:2 4 6
合并后链表C:1 2 3 4 5 6
```
阅读全文
相关推荐
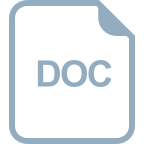
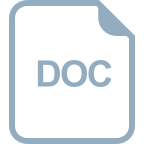
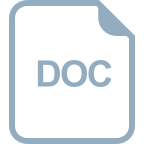















