这段报错了priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
时间: 2023-07-09 15:06:42 浏览: 61
这段代码中使用了一个优先队列(priority_queue)容器,它的模板参数是一个pair<int, int>类型的元素,元素类型为int的第一个值作为优先级,第二个值作为队列中的元素。另外,我们还指定了一个vector作为底层容器,以及一个greater函数对象作为比较函数,表示使用从小到大的方式对元素进行排序。
这段代码的报错可能是因为你没有包含相应的头文件,可以尝试添加以下语句:
```c++
#include <queue> // 包含priority_queue容器的头文件
#include <vector> // 包含vector容器的头文件
using namespace std;
```
如果还是报错,可以提供具体的错误信息和上下文,以便更好地帮助你解决问题。
相关问题
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq
This is a declaration of a priority queue in C++ that stores pairs of integers. The priority queue is implemented using a min-heap, which means that the smallest element will have the highest priority. The first integer in the pair is the priority and the second integer is the value.
The syntax for declaring a priority queue in C++ is:
priority_queue<data_type, container_type, comparison_function>
In this case, the data type is a pair of integers (pair<int, int>), the container type is a vector (vector<pair<int, int>>), and the comparison function is the default less-than operator (>).
However, in order to implement a min-heap, we need to use the greater-than operator (<) instead. So we pass greater<pair<int, int>> as the comparison function.
Overall, this priority queue will store pairs of integers in ascending order of their first element. If two pairs have the same first element, then they will be ordered based on their second element in ascending order as well.
priority_queue定义greater<>
```C++
priority_queue<int, vector<int>, greater<int>> q;```
以上代码定义了一个最小值的优先队列,其中greater<int>是一个函数对象,用于比较两个元素的大小。在这种情况下,它将第二个参数与第一个参数进行比较,如果第二个参数小于第一个参数,则返回true。这意味着在队列中,元素按升序排序,即最小的元素在队列的前面。如果您想要定义一个最大值的优先队列,可以省略greater<int>,因为它是默认的比较函数对象。
对于priority_queue<pair<int,int>,vector<pair<int,int>>,greater<pair<int,int>>> q,存在有两种元素的排列顺序,即按照pair的第一个元素升序排序,如果第一个元素相同,则按照第二个元素升序排序。
相关推荐
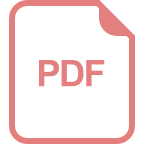
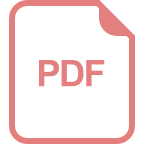
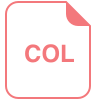
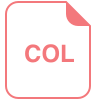
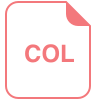









