在vue2中如何通过three.js构建一个简单的三维模型
时间: 2024-10-31 17:10:33 浏览: 12
在 Vue 2 中结合 Three.js 构建三维模型,你需要安装 `vue-threejs` 这个库,它为 Vue 提供了一个方便的方式来集成 Three.js 功能。以下是一个基本步骤:
1. **安装依赖**:
首先确保你已经安装了 Node.js 和 npm(如果还没有,可以从 https://nodejs.org/ 下载)。然后,在项目目录下运行:
```
npm install vue three @vue/three/dist/vue-three
```
2. **设置组件**:
创建一个新的 Vue 组件并引入 Three.js 的核心库:
```html
<template>
<div id="app">
<canvas ref="canvas"></canvas>
</div>
</template>
<script>
import * as THREE from 'three';
import { createCanvas, OrbitControls } from '@vue/three/dist/vue-three';
export default {
setup() {
const canvas = createCanvas({ attachTo: this.$refs.canvas });
const scene = new THREE.Scene();
// 更多的创建和配置...
return { canvas, scene };
},
};
</script>
```
3. **创建几何体**:
你可以创建一个简单的立方体作为模型基础:
```javascript
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
```
4. **添加相机和控制器**:
```javascript
const camera = new THREE.PerspectiveCamera(75, canvas.width / canvas.height, 0.1, 1000);
camera.position.z = 5; // 设置相机位置
const controls = new OrbitControls(camera, canvas.domElement);
controls.update(); // 更新控制
```
5. **渲染循环**:
使用 Vue 的生命周期钩子(如 `mounted()`)来定期更新场景:
```javascript
mounted() {
requestAnimationFrame(() => {
this.render();
requestAnimationFrame(this.mounted);
});
},
methods: {
render() {
// 渲染逻辑
renderer.render(scene, camera);
controls.update();
}
}
```
6. **导出组件**:
最后,把这个组件注册到你的应用中。
这是一个非常基础的开始,Three.js 提供了许多其他功能,比如光照、动画、纹理等。如果你需要更复杂的模型或交互,记得查阅官方文档:https://threejs.org/docs/
阅读全文
相关推荐
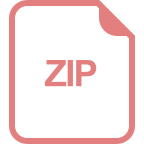
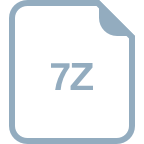
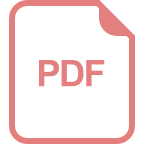















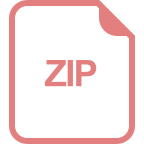