int main(void) { /* Initialize hardware */ HAL_BOARD_INIT(); // Initialize board I/O InitBoard( OB_COLD ); /* Initialze the HAL driver */ HalDriverInit(); /* Initialize NV system */ osal_snv_init(); /* Initialize LL */ /* Initialize the operating system */ osal_init_system(); /* Enable interrupts */ HAL_ENABLE_INTERRUPTS(); // Final board initialization InitBoard( OB_READY ); #if defined ( POWER_SAVING ) osal_pwrmgr_device( PWRMGR_BATTERY ); #endif /* Start OSAL */ osal_start_system(); // No Return from here return 0; }
时间: 2024-03-31 07:37:36 浏览: 175
这是一段 C 语言程序,它的作用是初始化硬件、驱动和操作系统,并启动操作系统。程序流程如下:
1. 初始化硬件,包括初始化板子的输入输出。
2. 初始化 HAL 驱动。
3. 初始化 NV 系统。
4. 初始化 LL。
5. 初始化操作系统。
6. 启用中断。
7. 完成最后的板子初始化。
8. 开启操作系统。
该程序中的一些函数和宏定义可能是针对特定的硬件平台和操作系统的,因此需要结合具体的开发环境来理解。
相关问题
基于stm32单片机hal库写出SGP30传感器的C文件和h文件
SGP30是一款用于测量环境中的VOCs(挥发性有机化合物)和TVOCs(总挥发性有机物)浓度的传感器。基于STM32单片机的HAL库(Hardware Abstraction Layer,硬件抽象层),我们可以创建专门的驱动程序来控制SGP30。这里提供一个简化的示例,假设你已经有了基本的HAL库配置。
首先,我们创建SGP30_driver.h头文件:
```c
// SGP30_driver.h
#ifndef SGP30_DRIVER_H
#define SGP30_DRIVER_H
#include "stm32f4xx_hal.h"
#include "hal_sgp30.h"
typedef enum {
SGP30_SUCCESS = 0,
SGP30_ERROR
} sgp30_error_t;
/**
* @brief Initialize SGP30 sensor with HAL
*/
sgp30_error_t sgp30_init(void);
/**
* @brief Read VOC and TVOC concentrations from SGP30
*
* @param[out] voc_result - Pointer to store VOC concentration in parts per billion (ppb)
* @param[out] tvoc_result - Pointer to store TVOC concentration in ppb
*/
void sgp30_read_data(float* voc_result, float* tvoc_result);
/**
* @brief Deinitialize the SGP30 sensor
*/
void sgp30_deinit(void);
#endif /* SGP30_DRIVER_H */
```
然后,在SGP30_driver.c文件里实现这些函数的功能:
```c
// SGP30_driver.c
#include "SGP30_driver.h"
#include "hal_sgp30.h"
sgp30_error_t sgp30_init() {
GPIO_InitTypeDef GPIO_InitStruct;
I2C_HandleTypeDef hi2c1;
// Initialize SGP30 pins and I2C communication
GPIO_InitStruct.Pin = GPIO_PIN_6 | GPIO_PIN_7; // GPIO for SGP30 I2C interface
GPIO_InitStruct.Mode = GPIO_MODE_AF_OD;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH;
GPIO_InitStruct.Alternate = GPIO_AF4_I2C1;
HAL_GPIO_Init(GPIOB, &GPIO_InitStruct);
hi2c1.Instance = I2C1;
hi2c1.Init.ClockSpeed = 400000; // Adjust this based on your board's I2C speed
hi2c1.Init.DutyCycle = I2C_DUTYCYCLE_2;
hi2c1.Init.OwnAddress1 = I2C_OA_NOADDR;
hi2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT;
hi2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE;
hi2c1.Init.OwnAddress2 = 0;
hi2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE;
hi2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE;
if (HAL_I2C_Init(&hi2c1) != HAL_OK) {
return SGP30_ERROR;
}
// Set up SGP30 registers (example only, see datasheet)
HAL_SGP30_Init();
return SGP30_SUCCESS;
}
void sgp30_read_data(float* voc_result, float* tvoc_result) {
uint8_t data[6];
HAL_StatusTypeDef status;
// Read SGP30 data, usually from specific registers
status = HAL_SGP30_ReadData(&hi2c1, data, sizeof(data));
if (status == HAL_OK) {
// Convert raw data into concentrations, assuming you have a conversion function
*voc_result = convert_sgp30_voc(data);
*tvoc_result = convert_sgp30_tvoc(data);
} else {
*voc_result = *tvoc_result = 0.0f; // Handle error or uninitialized state
}
}
void sgp30_deinit() {
HAL_GPIO_DeInit(GPIOB, GPIO_PIN_6 | GPIO_PIN_7); // Release I2C pins
HAL_I2C_DeInit(&hi2c1);
}
```
这个例子仅作参考,实际应用中需要根据具体的硬件连接、I2C通信设置以及SGP30的具体数据转换函数来调整代码。另外,别忘了检查是否已包含相应的HAL库,并确保STM32F4xx_HAL_H文件已经正确引入。
阅读全文
相关推荐
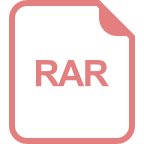
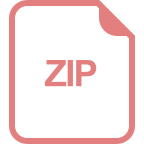
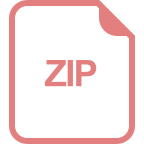
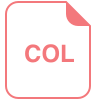
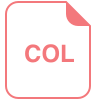
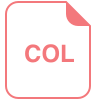
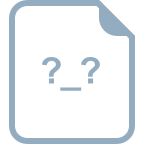
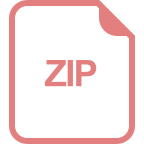
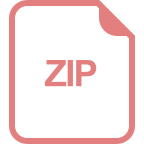
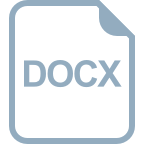
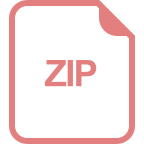
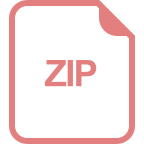
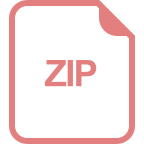
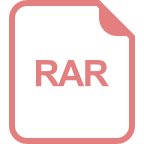