用c++对图片进行连通域分析判断连通域是否涉及图像的边
时间: 2023-05-18 15:06:00 浏览: 61
非常感谢您的提问。对于这个问题,可以使用OpenCV库中的函数来实现连通域分析。具体来说,可以使用cv::connectedComponents函数来计算图像中的连通域数量,并使用cv::findContours函数来查找每个连通域的轮廓。然后,可以使用cv::pointPolygonTest函数来检查每个轮廓是否与图像的边界相交。如果相交,则说明该连通域涉及图像的边缘。以下是示例代码:
```
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat image = imread("image.jpg", IMREAD_GRAYSCALE);
// Perform connected components analysis
Mat labels;
int num_components = connectedComponents(image, labels);
// Find contours for each component
std::vector<std::vector<Point>> contours(num_components);
for (int i = 0; i < image.rows; i++)
{
for (int j = 0; j < image.cols; j++)
{
int label = labels.at<int>(i, j);
if (label > 0)
{
contours[label].push_back(Point(j, i));
}
}
}
// Check if each contour intersects image boundary
for (int i = 1; i < num_components; i++)
{
if (pointPolygonTest(contours[i], Point(0, 0), false) >= 0 ||
pointPolygonTest(contours[i], Point(image.cols - 1, 0), false) >= 0 ||
pointPolygonTest(contours[i], Point(image.cols - 1, image.rows - 1), false) >= 0 ||
pointPolygonTest(contours[i], Point(0, image.rows - 1), false) >= 0)
{
std::cout << "Connected component " << i << " intersects image boundary." << std::endl;
}
}
return 0;
}
```
希望这个回答能够帮助到您!
相关推荐
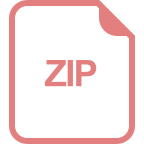














