从身份证中提取学生的性别年龄按今年2023年算年龄用c语言
时间: 2024-03-04 17:51:18 浏览: 121
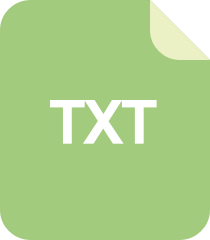
c#根据身份证计算性别 年龄
可以通过身份证号码中的出生日期来计算学生的年龄,而性别则可以通过身份证号码中的第17位数字来判断。具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <string.h>
#include <time.h>
int main() {
char id[19];
printf("请输入身份证号码:");
scanf("%s", id);
// 提取出生日期
char birth[9];
strncpy(birth, id + 6, 8);
birth[8] = '\0';
// 计算年龄
int birth_year = atoi(strncpy((char[5]){0}, birth, 4)) - 1900;
int birth_month = atoi(strncpy((char[3]){0}, birth + 4, 2)) - 1;
int birth_day = atoi(strncpy((char[3]){0}, birth + 6, 2));
struct tm birthday = {0};
birthday.tm_year = birth_year;
birthday.tm_mon = birth_month;
birthday.tm_mday = birth_day;
time_t birth_time = mktime(&birthday);
time_t now = time(NULL);
int age = (int)difftime(now, birth_time) / (365 * 24 * 60 * 60);
// 判断性别
char gender = id[16] % 2 == 0 ? 'F' : 'M';
printf("年龄:%d\n性别:%c\n", age, gender);
return 0;
}
```
上述代码中,首先通过 `scanf` 函数获取用户输入的身份证号码,并从中提取出生日期。然后使用 `mktime` 函数将出生日期转换成 `time_t` 类型,再通过 `difftime` 函数计算出年龄。最后通过判断身份证号码中的第17位数字的奇偶性来确定性别,并输出结果。需要注意的是,身份证号码中的出生日期和性别信息并不是按照固定位置存储的,而是根据标准进行编码的,因此需要对身份证号码进行解析才能得到正确的结果。
阅读全文
相关推荐


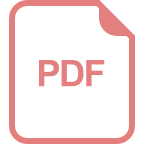
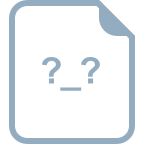
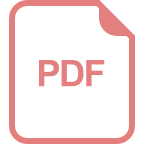
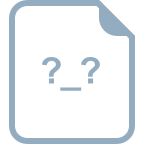
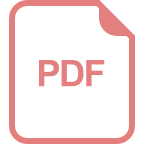
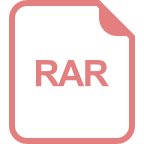
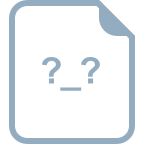