java如何实现post请求
时间: 2024-01-18 14:02:30 浏览: 45
在Java中实现POST请求,可以使用HttpURLConnection或者HttpClient两种方式。下面分别介绍这两种方式:
1. 使用HttpURLConnection实现POST请求:
```java
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpUrlConnectionPostDemo {
public static void main(String[] args) throws Exception {
URL url = new URL("http://example.com/api");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json;charset=UTF-8");
conn.setDoOutput(true);
String jsonInputString = "{\"username\": \"admin\",\"password\": \"password\"}";
try (OutputStream os = conn.getOutputStream()) {
byte[] input = jsonInputString.getBytes("utf-8");
os.write(input, 0, input.length);
}
try (BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream(), "utf-8"))) {
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
System.out.println(response.toString());
}
}
}
```
2. 使用HttpClient实现POST请求:
```java
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClientBuilder;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class HttpClientPostDemo {
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpClientBuilder.create().build();
HttpPost request = new HttpPost("http://example.com/api");
String jsonInputString = "{\"username\": \"admin\",\"password\": \"password\"}";
StringEntity params = new StringEntity(jsonInputString);
request.addHeader("content-type", "application/json");
request.setEntity(params);
HttpResponse response = httpClient.execute(request);
BufferedReader reader = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
StringBuilder result = new StringBuilder();
String line = "";
while ((line = reader.readLine()) != null) {
result.append(line);
}
System.out.println(result.toString());
}
}
```
这两种方式都可以实现POST请求,具体使用哪种方式取决于具体场景和个人喜好。
相关推荐
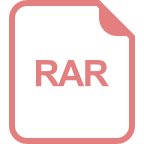
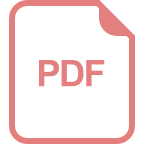
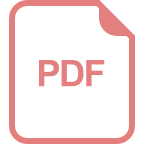
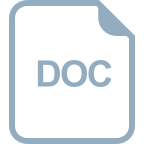
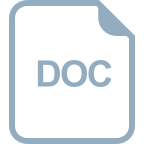
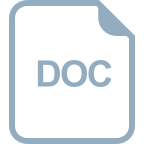
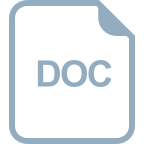
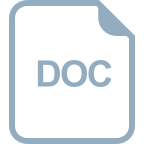
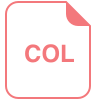
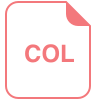
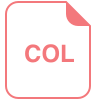
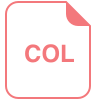
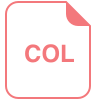




