pyhton编写一个函数,输入n为偶数时,调用函数求1/2+1/4+...+1/n,当输入n为奇数时,调用函数1/1+1/3+...+1/n
时间: 2023-06-01 18:05:25 浏览: 112
好的,我理解了你的请求。以下是Python编写的函数,以实现你所要求的功能:
```python
def calculate_fraction(n):
if n % 2 == 0:
# 当n为偶数时
result = 0
for i in range(2, n+1, 2):
result += 1 / i
return result
else:
# 当n为奇数时
result = 0
for i in range(1, n+1, 2):
result += 1 / i
return result
```
当你输入一个偶数n时,该函数将返回1/2 + 1/4 + ... + 1/n的总和,当你输入一个奇数n时,该函数将返回1/1 + 1/3 + ... + 1/n的总和。希望这个函数可以满足你的需求。
相关问题
请帮我用pyhton编写程序,分别输入3个字符串,依次验证其是否为有效的电话号码、邮政编码和网站网址格式。 (1)电话号码必须是8位号码,如果有区号,区号必须是3位。 (2)邮政编码必须是6位数字。 (3)网站网址的正则表示参考:r'^https?://\w+(?:.[^.]+)+(?:/.+)*$'
import re
# 验证电话号码
def check_phone_number(phone_number):
pattern = r'^\d{8}$|^\d{3}\-\d{8}$'
if re.match(pattern, phone_number):
return True
else:
return False
# 验证邮政编码
def check_postal_code(postal_code):
pattern = r'^\d{6}$'
if re.match(pattern, postal_code):
return True
else:
return False
# 验证网站网址
def check_website(website):
pattern = r'^https?://\w+(?:\.[^.\s]+)*(?:/\S+)*$'
if re.match(pattern, website):
return True
else:
return False
# 输入电话号码、邮政编码、网站网址
phone_number = input("请输入电话号码:")
postal_code = input("请输入邮政编码:")
website = input("请输入网站网址:")
# 验证并输出结果
if check_phone_number(phone_number):
print("电话号码是有效的。")
else:
print("电话号码不是有效的。")
if check_postal_code(postal_code):
print("邮政编码是有效的。")
else:
print("邮政编码不是有效的。")
if check_website(website):
print("网站网址是有效的。")
else:
print("网站网址不是有效的。")
pyhton 爬取https://bz.feigua.cn/ranking/DailyHotVideoV2/20230725/1/0.html 页面数据
你可以使用 Python 的 requests 和 BeautifulSoup 库来爬取网页数据。以下是一个示例代码,可以帮助你实现这个任务:
```python
import requests
from bs4 import BeautifulSoup
url = "https://bz.feigua.cn/ranking/DailyHotVideoV2/20230725/1/0.html"
# 发送GET请求获取页面内容
response = requests.get(url)
# 使用BeautifulSoup解析页面内容
soup = BeautifulSoup(response.text, "html.parser")
# 找到需要的数据
data = soup.find_all("div", class_="video-item") # 假设需要获取视频项的数据
# 处理数据
for item in data:
# 进行数据提取和处理
# ...
# 输出结果
# ...
```
你需要根据需要提取的具体数据,使用适当的标签和类名来修改代码中的 `find_all` 方法的参数。此外,还可以使用其他方法和工具来处理网页数据,比如使用正则表达式、XPath等。
请注意,网站的所有者可能有反爬虫机制,你可能需要处理一些反爬虫措施,如添加请求头、处理验证码等。在编写爬虫时,请遵守网站的爬虫规则,并确保自己的行为合法合规。
阅读全文
相关推荐
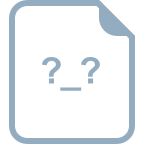













