java取两个int数组的交集和异或集
时间: 2024-02-21 14:59:09 浏览: 150
可以使用Java中的Set来实现两个int数组的交集和异或集。
首先,我们可以使用HashSet来创建一个int数组的Set,并使用addAll方法将第一个数组A中的元素添加到Set中。接着,我们可以使用retainAll方法来保留Set中同时存在于第一个数组A和第二个数组B中的元素,即得到两个数组的交集:
```java
int[] A = {1, 2, 3};
int[] B = {2, 3, 4};
Set<Integer> setA = new HashSet<>();
for (int i : A) setA.add(i);
Set<Integer> setB = new HashSet<>();
for (int i : B) setB.add(i);
Set<Integer> intersection = new HashSet<>(setA);
intersection.retainAll(setB);
```
得到的intersection即为两个数组的交集。
接着,我们可以使用Set的addAll方法将两个数组中的元素都添加到Set中,即得到两个数组的并集。接着,我们可以使用Set的removeAll方法将两个数组的交集从Set中移除,即得到两个数组的异或集:
```java
Set<Integer> union = new HashSet<>(setA);
union.addAll(setB);
Set<Integer> xorSet = new HashSet<>(union);
xorSet.removeAll(intersection);
```
得到的xorSet即为两个数组的异或集。最后,我们可以将Set转换为int数组:
```java
int[] intersectionArray = intersection.stream().mapToInt(Integer::intValue).toArray();
int[] xorSetArray = xorSet.stream().mapToInt(Integer::intValue).toArray();
```
阅读全文
相关推荐


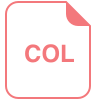
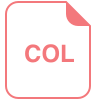
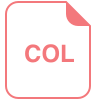

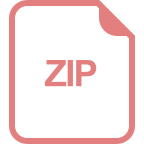
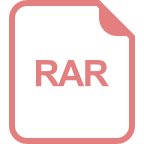
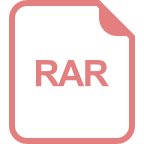