vue3组件导出了方法,用ref获取值然后获取值为undefind
时间: 2024-02-21 13:58:40 浏览: 191
在 Vue3 中,如果你在组件中导出了一个方法,并且在使用该方法时,你使用了 ref 获取其返回值,却发现该值为 undefined,那么可能有以下一些原因:
1. 方法没有返回一个 ref 对象
在 Vue3 中,如果你想要通过 ref 获取一个数据的值,你必须将该数据封装到一个 ref 对象中。如果你的方法没有正确地返回一个 ref 对象,那么在使用 ref 时获取的值就会是 undefined。
例如,下面的代码中,myMethod 只是返回了一个普通的对象,而没有将其封装到一个 ref 对象中:
```
// MyComponent.vue
<script>
export const myMethod = () => {
return { name: 'John' };
};
</script>
```
在另一个组件中使用 ref 来获取 myMethod 的返回值时,你会发现该值为 undefined:
```
// AnotherComponent.vue
<template>
<div>{{ myName }}</div>
</template>
<script>
import { myMethod } from './MyComponent';
export default {
setup() {
const myRef = myMethod();
const myName = ref(myRef.value.name);
return { myName };
}
};
</script>
```
要解决这个问题,你需要将 myMethod 的返回值封装到一个 ref 对象中。例如:
```
// MyComponent.vue
<script>
export const myMethod = () => {
const name = ref('John');
return { name };
};
</script>
```
2. ref 对象的 value 属性被修改了
如果你的方法返回了一个正确的 ref 对象,但在使用 ref 时获取的值仍然是 undefined,那么可能是因为 ref 对象的 value 属性被修改了。
在 Vue3 中,如果你想要修改一个 ref 对象的值,你需要使用 `.value` 来访问它的 value 属性。如果你直接修改了 ref 对象本身,那么在使用 ref 时获取的值就会是 undefined。
例如,下面的代码中,myMethod 返回了一个封装了 name 数据的 ref 对象。但在使用 ref 时获取 name 的值时,我们直接修改了该 ref 对象本身,而没有通过 .value 来访问它的 value 属性:
```
// MyComponent.vue
<script>
export const myMethod = () => {
const name = ref('John');
name = 'Tom'; // 直接修改 ref 对象本身,而不是访问它的 value 属性
return { name };
};
</script>
```
在另一个组件中使用 ref 来获取 myMethod 的返回值时,你会发现该值为 undefined:
```
// AnotherComponent.vue
<template>
<div>{{ myName }}</div>
</template>
<script>
import { myMethod } from './MyComponent';
export default {
setup() {
const myRef = myMethod();
const myName = ref(myRef.value.name);
return { myName };
}
};
</script>
```
要解决这个问题,你需要通过 `.value` 来访问 ref 对象的 value 属性,并且不要直接修改 ref 对象本身。例如:
```
// MyComponent.vue
<script>
export const myMethod = () => {
const name = ref('John');
name.value = 'Tom'; // 通过 .value 来访问 value 属性,而不是直接修改 ref 对象本身
return { name };
};
</script>
```
阅读全文
相关推荐
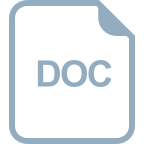
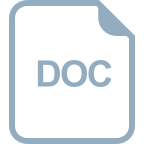
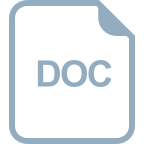

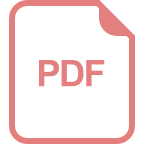
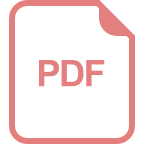
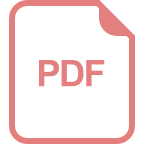
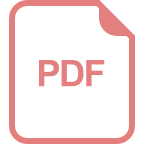
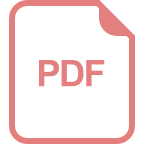
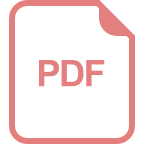








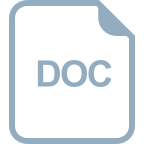