mockMvc 模拟get请求 500
时间: 2023-11-22 12:35:54 浏览: 32
出现500错误可能有多种原因,以下是一些可能导致500错误的原因及解决方法:
1. 参数错误:请确保你向模拟的get请求中提供了正确的参数,如果参数有误,服务器就会返回500错误。
2. 接口错误:请确保你模拟的get请求的接口地址是正确的,如果接口地址有误,服务器就会返回500错误。
3. 代码错误:请确保你的代码没有语法错误或逻辑错误,如果代码有误,服务器就会返回500错误。
4. 服务器错误:请确保你的服务器正常运行,如果服务器出现故障,就会导致500错误。
如果你无法通过以上方法解决问题,建议你查看服务器的日志文件,以了解更多有关500错误的信息。另外,你也可以尝试使用其他工具模拟get请求,以确认问题是否出现在mockMvc中。
相关问题
mockmvc 模拟xml接口
您可以使用MockMvc框架来模拟XML接口。这是一个示例代码:
```java
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
import static org.springframework.test.web.servlet.setup.MockMvcBuilders.*;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.http.MediaType;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.MvcResult;
@RunWith(SpringRunner.class)
@WebMvcTest(XmlController.class)
public class XmlControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetXml() throws Exception {
MvcResult result = mockMvc.perform(get("/xml")
.accept(MediaType.APPLICATION_XML))
.andExpect(status().isOk())
.andReturn();
String xmlResponse = result.getResponse().getContentAsString();
// Do your assertions on the XML response
}
}
```
在这个例子中,我们使用MockMvc来模拟一个GET请求到"/xml"端点,并且指定接受的媒体类型为"application/xml"。然后,我们使用MockMvcResultMatchers来断言返回的状态码为200。最后,我们可以使用MvcResult对象来获取XML响应,并在其中进行必要的断言。
springboot单测MockMvc设置请求头
在 Spring Boot 单元测试中,可以使用 `MockMvc` 对象模拟 HTTP 请求,并设置请求头。下面是一个示例代码:
```java
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.http.MediaType;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.result.MockMvcResultHandlers;
import org.springframework.test.web.servlet.result.MockMvcResultMatchers;
@WebMvcTest(YourController.class)
public class YourControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testWithCustomHeaders() throws Exception {
mockMvc.perform(MockMvcRequestBuilders.get("/your-endpoint")
.header("X-Custom-Header", "value")
.accept(MediaType.APPLICATION_JSON))
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(MockMvcResultHandlers.print());
}
}
```
在上面的示例中,我们使用 `MockMvc` 执行一个 GET 请求,并设置了一个自定义的请求头 `X-Custom-Header` 的值为 `"value"`。同时,我们还设置了 Accept 头部为 `"application/json"`。
确保替换示例中的 `/your-endpoint` 为你要测试的端点路径,并根据需要更改请求方法(`get()`、`post()` 等)和其他请求配置。
相关推荐
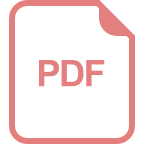
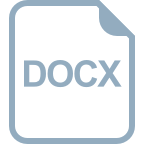
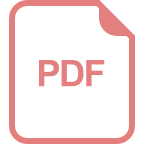












