设计一个 StudentInfo 类,它能保存: (1)学生名字; (2)学号,格式为定长的10个字符; (3)专业名; 自行设计相应成员函数。 再设计一个 Grades 类,继承上述类,它能保存:考试成绩,共六个科目。 它有一个成员函数,getAverage,返回六科平均成绩。 自行设计另外的相应成员函数。 编程任务: (1)定义一个 Grades 类的对象数组,长度为 3,用户依次输入学生信息,保存到对象数组当中,最后按平均分数从高到低,依次输出同学的个人信息和平均分。 (2)检测输入有效性,保证输入的成绩是 [0, 100] 的实数。 (3)要求使用多文件结构c
时间: 2024-04-28 16:21:21 浏览: 121
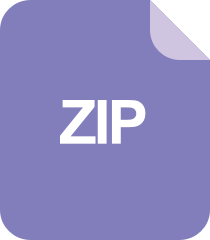
StudentInfo:一个学生信息查询和微信信息的demo
为了实现这个任务,我们需要将类的声明和定义分别写在不同的文件中。下面是实现这个任务的代码示例:
**StudentInfo.h:**
```cpp
#ifndef STUDENTINFO_H
#define STUDENTINFO_H
#include <string>
class StudentInfo {
public:
StudentInfo();
StudentInfo(const std::string& name, const std::string& id, const std::string& major);
void setName(const std::string& name);
void setId(const std::string& id);
void setMajor(const std::string& major);
std::string getName() const;
std::string getId() const;
std::string getMajor() const;
private:
std::string name;
std::string id;
std::string major;
};
#endif // STUDENTINFO_H
```
**StudentInfo.cpp:**
```cpp
#include "StudentInfo.h"
StudentInfo::StudentInfo() {}
StudentInfo::StudentInfo(const std::string& name, const std::string& id, const std::string& major)
: name(name), id(id), major(major) {}
void StudentInfo::setName(const std::string& name) {
this->name = name;
}
void StudentInfo::setId(const std::string& id) {
this->id = id;
}
void StudentInfo::setMajor(const std::string& major) {
this->major = major;
}
std::string StudentInfo::getName() const {
return name;
}
std::string StudentInfo::getId() const {
return id;
}
std::string StudentInfo::getMajor() const {
return major;
}
```
**Grades.h:**
```cpp
#ifndef GRADES_H
#define GRADES_H
#include "StudentInfo.h"
class Grades : public StudentInfo {
public:
Grades();
Grades(const std::string& name, const std::string& id, const std::string& major,
double grade1, double grade2, double grade3, double grade4, double grade5, double grade6);
void setGrades(double grade1, double grade2, double grade3, double grade4, double grade5, double grade6);
double getAverage() const;
private:
double grades[6];
};
#endif // GRADES_H
```
**Grades.cpp:**
```cpp
#include "Grades.h"
Grades::Grades() {}
Grades::Grades(const std::string& name, const std::string& id, const std::string& major,
double grade1, double grade2, double grade3, double grade4, double grade5, double grade6)
: StudentInfo(name, id, major), grades{grade1, grade2, grade3, grade4, grade5, grade6} {}
void Grades::setGrades(double grade1, double grade2, double grade3, double grade4, double grade5, double grade6) {
grades[0] = grade1;
grades[1] = grade2;
grades[2] = grade3;
grades[3] = grade4;
grades[4] = grade5;
grades[5] = grade6;
}
double Grades::getAverage() const {
double sum = 0;
for (int i = 0; i < 6; i++) {
sum += grades[i];
}
return sum / 6;
}
```
**main.cpp:**
```cpp
#include "Grades.h"
#include <iostream>
#include <algorithm>
int main() {
Grades students[3];
for (int i = 0; i < 3; i++) {
std::string name, id, major;
double grades[6];
std::cout << "Please enter student's name: ";
std::getline(std::cin, name);
students[i].setName(name);
std::cout << "Please enter student's ID: ";
std::getline(std::cin, id);
students[i].setId(id);
std::cout << "Please enter student's major: ";
std::getline(std::cin, major);
students[i].setMajor(major);
std::cout << "Please enter the grades for six courses: ";
std::cin >> grades[0] >> grades[1] >> grades[2] >> grades[3] >> grades[4] >> grades[5];
// Check input validity
while (std::cin.fail() || std::any_of(grades, grades + 6, [](double grade){return grade < 0 || grade > 100;})) {
std::cout << "Invalid input! Please enter the grades again: ";
std::cin.clear();
std::cin.ignore(10000, '\n');
std::cin >> grades[0] >> grades[1] >> grades[2] >> grades[3] >> grades[4] >> grades[5];
}
students[i].setGrades(grades[0], grades[1], grades[2], grades[3], grades[4], grades[5]);
std::cin.ignore(10000, '\n');
}
// Sort students by average grades
std::sort(students, students + 3, [](const Grades& s1, const Grades& s2){return s1.getAverage() > s2.getAverage();});
// Output students' information
for (int i = 0; i < 3; i++) {
std::cout << "Name: " << students[i].getName() << std::endl;
std::cout << "ID: " << students[i].getId() << std::endl;
std::cout << "Major: " << students[i].getMajor() << std::endl;
std::cout << "Average grade: " << students[i].getAverage() << std::endl << std::endl;
}
return 0;
}
```
注意,在编写多文件结构程序时,需要先编译每个文件,然后将它们链接起来。假设我们的文件名是 StudentInfo.cpp、Grades.cpp 和 main.cpp,我们可以使用以下命令编译和链接:
```
$ g++ -c StudentInfo.cpp
$ g++ -c Grades.cpp
$ g++ -c main.cpp
$ g++ -o program main.o StudentInfo.o Grades.o
$ ./program
```
阅读全文
相关推荐
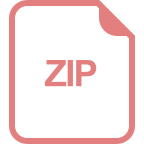
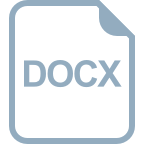











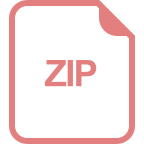
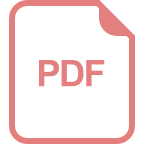
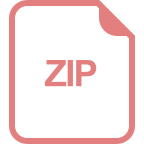