pandas matplotlib example
时间: 2023-09-06 21:11:07 浏览: 51
Here's an example of using pandas and matplotlib to plot a line graph:
```
import pandas as pd
import matplotlib.pyplot as plt
# create a dataframe with some sample data
data = {'year': [2010, 2011, 2012, 2013, 2014, 2015, 2016],
'sales': [100, 120, 140, 160, 180, 200, 220]}
df = pd.DataFrame(data)
# plot the data as a line graph
plt.plot(df['year'], df['sales'])
# add labels and title
plt.xlabel('Year')
plt.ylabel('Sales')
plt.title('Sales over Time')
# display the plot
plt.show()
```
This will create a line graph of sales over time, with the year on the x-axis and the sales on the y-axis. The plot will have labels and a title, and will be displayed using matplotlib.
相关问题
用到requests、BeautifulSoup、pandas和matplotlib
好的,你需要使用 requests 库来请求网页数据,使用 BeautifulSoup 库来解析网页数据,使用 pandas 库来处理数据,使用 matplotlib 库来绘制数据图表。以下是一个简单的例子:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
import matplotlib.pyplot as plt
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
# 从网页中提取数据
data = []
table = soup.find("table")
rows = table.find_all("tr")
for row in rows:
cols = row.find_all("td")
cols = [col.text.strip() for col in cols]
data.append(cols)
# 将数据保存为 CSV 文件
df = pd.DataFrame(data, columns=["列1", "列2", "列3"])
df.to_csv("data.csv", index=False)
# 绘制数据图表
plt.plot(df["列1"], df["列2"])
plt.xlabel("X轴标签")
plt.ylabel("Y轴标签")
plt.title("标题")
plt.show()
```
你可以根据自己的需求修改代码,例如修改 URL 、解析 HTML 的方式、处理数据的方式、绘制图表的样式等。
matplotlib用途
Matplotlib是一个Python的2D绘图库,它可以用来创建各种静态,动态,交互式的图表、图形和可视化界面。Matplotlib可以用于数据可视化、科学计算、工程绘图、统计分析、金融分析等领域。它支持多种操作系统和图形输出格式,包括PNG、PDF、SVG、EPS等。Matplotlib还可以与NumPy、SciPy、Pandas等Python库一起使用,使得数据分析和可视化更加方便和高效。
以下是一个简单的Matplotlib绘图例子,用于绘制一条直线:
```python
import matplotlib.pyplot as plt
import numpy as np
x = np.arange(0, 10, 0.1)
y = x * 2
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Matplotlib Example')
plt.show()
```
相关推荐
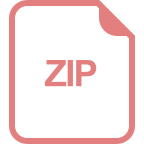
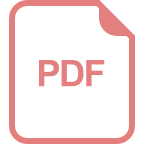
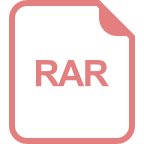












