帮我用c和pcap写一个能捕获数据包(可选择捕获离线还是在线和捕获的个数)、过滤数据包、保存数据包、分析数据包(要能和wireshark能够对比)(解析TCP协议、解析arp协议、解析udp协议、解析IP协议、解析icmp协议)
时间: 2023-08-05 08:02:06 浏览: 61
好的,我会尽力帮助你实现这个功能。首先,你需要安装libpcap库来处理数据包捕获。然后,你可以使用以下代码来实现捕获数据包和过滤数据包:
```c
#include <pcap.h>
#include <stdio.h>
#include <stdlib.h>
#include <netinet/in.h>
#include <arpa/inet.h>
void packet_handler(u_char *param, const struct pcap_pkthdr *header, const u_char *pkt_data);
int main(int argc, char **argv)
{
pcap_t *handle;
char errbuf[PCAP_ERRBUF_SIZE];
struct bpf_program fp;
char filter_exp[] = "tcp";
bpf_u_int32 net, mask;
int num_packets = 10;
if (argc < 2) {
printf("Usage: %s <interface>\n", argv[0]);
return 1;
}
handle = pcap_open_live(argv[1], BUFSIZ, 1, 1000, errbuf);
if (handle == NULL) {
printf("pcap_open_live() failed: %s\n", errbuf);
return 1;
}
if (pcap_lookupnet(argv[1], &net, &mask, errbuf) == -1) {
printf("pcap_lookupnet() failed: %s\n", errbuf);
return 1;
}
if (pcap_compile(handle, &fp, filter_exp, 0, net) == -1) {
printf("pcap_compile() failed\n");
return 1;
}
if (pcap_setfilter(handle, &fp) == -1) {
printf("pcap_setfilter() failed\n");
return 1;
}
pcap_loop(handle, num_packets, packet_handler, NULL);
pcap_close(handle);
return 0;
}
void packet_handler(u_char *param, const struct pcap_pkthdr *header, const u_char *pkt_data)
{
printf("Packet length: %d\n", header->len);
// 这里可以添加解析数据包的代码
}
```
这个程序可以捕获指定接口上的TCP数据包,你可以通过修改filter_exp变量来修改过滤规则,通过修改num_packets变量来修改捕获数据包的个数。
接下来,你需要使用pcap_dump_open和pcap_dump函数来保存捕获到的数据包:
```c
pcap_dumper_t *dumpfile;
char dumpfile_name[] = "capture.pcap";
dumpfile = pcap_dump_open(handle, dumpfile_name);
if (dumpfile == NULL) {
printf("pcap_dump_open() failed\n");
return 1;
}
pcap_loop(handle, num_packets, packet_handler, (u_char *)dumpfile);
pcap_dump_close(dumpfile);
```
最后,你需要编写解析数据包的代码来分析数据包。这可能需要一些复杂的结构体和位运算,但你可以参考Wireshark的源代码来理解协议的解析过程。
相关推荐
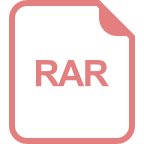
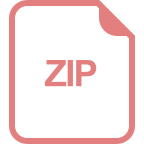
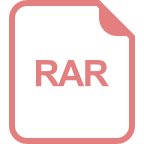
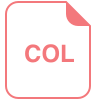
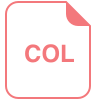
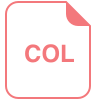
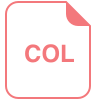
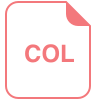









