写一个用libpcap捕获并保存数据包的代码
时间: 2024-02-24 13:57:01 浏览: 64
好的,以下是一个简单的使用libpcap捕获并保存数据包的C++代码:
```c++
#include <iostream>
#include <pcap.h>
void packet_handler(u_char* user_args, const struct pcap_pkthdr* packet_header, const u_char* packet_data) {
static int count = 1;
std::cout << "Packet #" << count << std::endl;
std::cout << "Packet size: " << packet_header->len << " bytes" << std::endl;
// 将数据包写入文件
FILE* fp;
fp = fopen("packets.pcap", "a");
if (fp != NULL) {
fwrite(packet_data, packet_header->len, 1, fp);
fclose(fp);
} else {
std::cerr << "Error opening file for writing packets" << std::endl;
}
count++;
}
int main() {
char errbuf[PCAP_ERRBUF_SIZE];
pcap_t* pcap_handle;
struct bpf_program filter;
char filter_exp[] = "ip";
bpf_u_int32 subnet_mask, ip;
// 打开网络接口
pcap_handle = pcap_open_live("eth0", BUFSIZ, 1, 1000, errbuf);
if (pcap_handle == NULL) {
std::cerr << "Error opening device: " << errbuf << std::endl;
return -1;
}
// 获取子网掩码和IP地址
if (pcap_lookupnet("eth0", &ip, &subnet_mask, errbuf) == -1) {
std::cerr << "Error getting IP/Subnet mask: " << errbuf << std::endl;
return -1;
}
// 编译过滤器
if (pcap_compile(pcap_handle, &filter, filter_exp, 0, subnet_mask) == -1) {
std::cerr << "Error compiling filter: " << pcap_geterr(pcap_handle) << std::endl;
return -1;
}
// 应用过滤器
if (pcap_setfilter(pcap_handle, &filter) == -1) {
std::cerr << "Error applying filter: " << pcap_geterr(pcap_handle) << std::endl;
return -1;
}
// 循环捕获数据包
pcap_loop(pcap_handle, -1, packet_handler, NULL);
// 关闭网络接口
pcap_close(pcap_handle);
return 0;
}
```
这段代码会使用libpcap库打开名为"eth0"的网络接口,并将捕获到的数据包保存到名为"packets.pcap"的文件中。在每次捕获到数据包时,它会在控制台输出数据包的编号和大小并将数据包写入文件。同时,它还会应用一个过滤器,只捕获IP数据包。
阅读全文
相关推荐
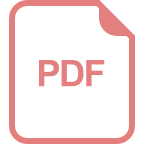
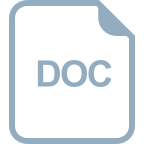
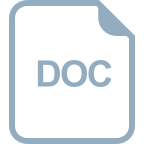
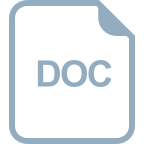
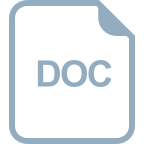



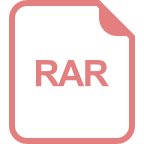
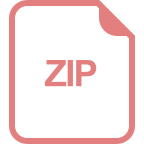
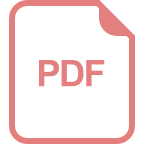
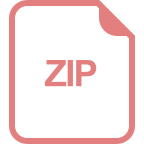
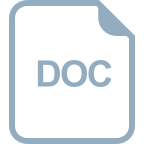
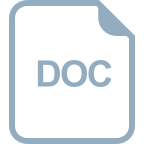
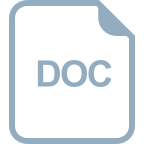