自定义类mystring实现运算符=重载
时间: 2023-11-10 07:02:05 浏览: 106
好的,可以参考下面的示例代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class mystring {
private:
char* str;
public:
mystring() {
str = NULL;
}
mystring(const char* s) {
int len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
}
mystring(const mystring& other) {
int len = strlen(other.str);
str = new char[len + 1];
strcpy(str, other.str);
}
~mystring() {
if (str != NULL) {
delete[] str;
str = NULL;
}
}
mystring& operator=(const mystring& other) {
if (this != &other) {
if (str != NULL) {
delete[] str;
str = NULL;
}
int len = strlen(other.str);
str = new char[len + 1];
strcpy(str, other.str);
}
return *this;
}
friend ostream& operator<<(ostream& os, const mystring& s) {
os << s.str;
return os;
}
};
int main() {
mystring s1("Hello");
mystring s2 = "World";
mystring s3(s1);
cout << "s1: " << s1 << endl;
cout << "s2: " << s2 << endl;
cout << "s3: " << s3 << endl;
s2 = s1;
cout << "s2: " << s2 << endl;
return 0;
}
```
在上面的代码中,我们自定义了一个字符串类 `mystring`,并实现了运算符 `=` 的重载。在 `operator=` 函数中,我们首先判断是否为自赋值,如果不是,就先释放原有的内存空间,再申请新的内存空间,并将字符串拷贝到新的内存中。
在 `main` 函数中,我们创建了三个 `mystring` 类型的对象 `s1`、`s2` 和 `s3`,并通过重载的 `<<` 运算符输出它们的值。然后将 `s1` 赋值给 `s2`,并再次输出 `s2` 的值,可以看到 `s2` 的值已经变成了 `Hello`。
阅读全文
相关推荐
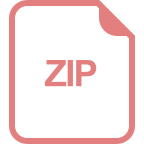


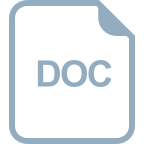
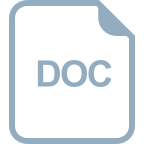
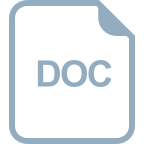
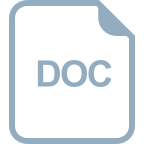

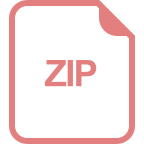
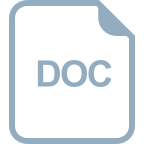
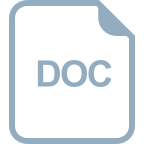
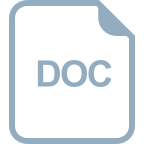
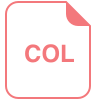



