声明一个字符串类string,用来存放不定长的字符串,重载运算符“==”,“<”和“>”,用于两个字符串的等于、小于和大于的比较运算。c++
时间: 2024-12-02 12:15:53 浏览: 13
在C++中,你可以通过在`std::basic_string`模板上自定义并实现运算符重载,来创建一个名为`string`的类,这个类将存储动态长度的字符串,并支持`==`(等于)、`<`(小于)和`>`(大于)的操作。下面是一个简单的例子,展示了如何重载这些运算符:
```cpp
#include <iostream>
#include <string>
class MyString : public std::string {
public:
// 重载"=="运算符,检查两个字符串是否相等
bool operator==(const MyString& other) const {
return *this == other;
}
// 重载"<"运算符,按字典顺序比较字符串
bool operator<(const MyString& other) const {
return *this < other;
}
// 重载">"运算符,同样的道理,但这里是按字典顺序逆向比较
bool operator>(const MyString& other) const {
return !(*this < other);
}
};
int main() {
MyString str1 = "hello";
MyString str2 = "world";
MyString str3 = "hello";
std::cout << "str1 == str2: " << (str1 == str2) << std::endl;
std::cout << "str1 < str2: " << (str1 < str2) << std::endl;
std::cout << "str1 > str2: " << (str1 > str2) << std::endl;
std::cout << "str1 == str3: " << (str1 == str3) << std::endl;
return 0;
}
```
在这个例子中,我们创建了一个`MyString`类,它继承自`std::string`,然后重写了`==`, `<`, 和 `>` 运算符。注意,`operator<` 实现时直接调用了 `*this < other`,而 `operator>` 则是通过取反 `!(*this < other)` 来得到相反的比较结果。
阅读全文
相关推荐
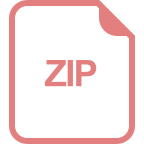
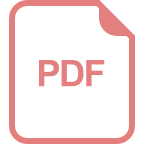
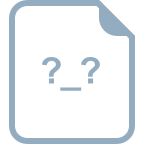




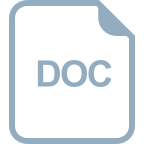
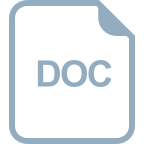
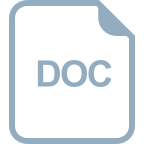
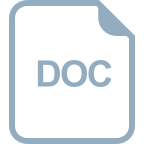
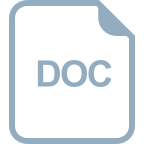
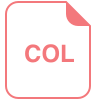
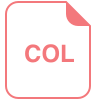
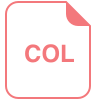
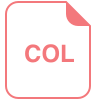

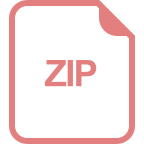
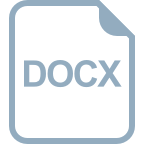