软中断通信 使用系统调用fork( )创建2个子进程p1和p2,在父进程中使用系统调用signal( )捕捉来自键盘上的软中断信号SIGINT(即按Ctrl-C),当捕捉到软中断信号SIGINT后,父进程使用系统调用kill( )分别向2个子进程发出软中断信号SIGUSR1和SIGUSR2,子进程捕捉到信号后分别输出信息“Child process p1 is killed by parent!”和“Child process p2 is killed by parent!”后终止。而父进程等待2个子进程终止后,输出信息“Parent process is killed!”后终止。
时间: 2023-06-19 08:10:21 浏览: 83
下面是使用软中断通信的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
void p1_handler(int sig) {
printf("Child process p1 is killed by parent!\n");
exit(0);
}
void p2_handler(int sig) {
printf("Child process p2 is killed by parent!\n");
exit(0);
}
int main() {
pid_t p1, p2;
int status;
// 创建子进程 p1
p1 = fork();
if (p1 < 0) {
perror("fork");
exit(1);
} else if (p1 == 0) {
// 子进程 p1
signal(SIGUSR1, p1_handler);
while(1);
}
// 创建子进程 p2
p2 = fork();
if (p2 < 0) {
perror("fork");
exit(1);
} else if (p2 == 0) {
// 子进程 p2
signal(SIGUSR2, p2_handler);
while(1);
}
// 父进程
signal(SIGINT, [](int sig){
kill(p1, SIGUSR1);
kill(p2, SIGUSR2);
});
// 等待子进程终止
waitpid(p1, &status, 0);
waitpid(p2, &status, 0);
printf("Parent process is killed!\n");
return 0;
}
```
在程序中,我们使用了 `signal` 函数来捕捉软中断信号 `SIGINT`,并在捕捉到信号后使用 `kill` 函数向子进程 p1 和 p2 发送信号 `SIGUSR1` 和 `SIGUSR2`。
子进程 p1 和 p2 分别使用 `signal` 函数来捕捉信号 `SIGUSR1` 和 `SIGUSR2`,并在收到信号后输出信息并使用 `exit` 函数退出。
父进程使用 `waitpid` 函数等待子进程终止,待子进程都终止后输出信息并使用 `exit` 函数退出。
相关推荐
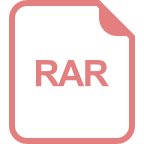
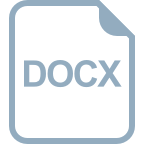
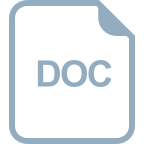














