使用pycharm 将多个excel放到一个excel的不同sheet里
时间: 2024-01-24 17:02:50 浏览: 113
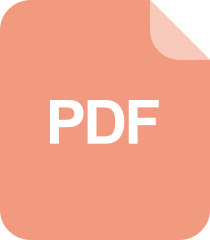
Python使用Excel将数据写入多个sheet
可以使用python中的pandas库来实现将多个excel文件合并到一个excel的不同sheet里。
以下是实现的步骤:
1. 导入pandas库和os库
```python
import pandas as pd
import os
```
2. 定义要合并的excel文件所在的文件夹路径
```python
folder_path = "path/to/excels/folder"
```
3. 获取文件夹中所有excel文件的文件名
```python
files = os.listdir(folder_path)
excel_files = [f for f in files if f.endswith('.xlsx')]
```
4. 定义一个空的pandas dataframe,用于存储所有excel文件的数据
```python
all_data = pd.DataFrame()
```
5. 循环遍历所有excel文件,将每个文件的数据添加到all_data中
```python
for file in excel_files:
full_path = os.path.join(folder_path, file)
data = pd.read_excel(full_path)
all_data = all_data.append(data, ignore_index=True)
```
6. 将all_data中的数据按照需要的方式分组,并将每个组的数据保存到一个新的excel文件的不同sheet里
```python
grouped_data = all_data.groupby('group_column_name')
with pd.ExcelWriter('output_file.xlsx') as writer:
for name, group in grouped_data:
group.to_excel(writer, sheet_name=name, index=False)
```
其中,'group_column_name'是用于分组的列的名称,'output_file.xlsx'是输出的excel文件的名称。
完整代码示例:
```python
import pandas as pd
import os
folder_path = "path/to/excels/folder"
files = os.listdir(folder_path)
excel_files = [f for f in files if f.endswith('.xlsx')]
all_data = pd.DataFrame()
for file in excel_files:
full_path = os.path.join(folder_path, file)
data = pd.read_excel(full_path)
all_data = all_data.append(data, ignore_index=True)
grouped_data = all_data.groupby('group_column_name')
with pd.ExcelWriter('output_file.xlsx') as writer:
for name, group in grouped_data:
group.to_excel(writer, sheet_name=name, index=False)
```
阅读全文
相关推荐















