写一段查找指定内容的Python程序
时间: 2023-02-26 21:26:10 浏览: 70
答:def find_content(list, content):
for i in list:
if i == content:
return i
return None
# 使用示例
list = [1, 2, 3, 5, 8]
content = 3
result = find_content(list, content)
print(result) # 输出3
相关问题
使用python读取程序的内存并查找指定字符
你可以使用 `psutil` 库来读取程序的内存,并通过遍历内存来查找指定字符。以下是一个示例代码:
```python
import psutil
def find_character(process_name, target_char):
for proc in psutil.process_iter(['name', 'memory_info']):
if proc.info['name'] == process_name:
with open(f"/proc/{proc.pid}/mem", 'rb') as f:
try:
mem_data = f.read()
if target_char.encode() in mem_data:
return True
except (PermissionError, FileNotFoundError):
pass
return False
process_name = "your_program.exe"
target_char = "X"
found = find_character(process_name, target_char)
if found:
print(f"Found '{target_char}' in memory of {process_name}.")
else:
print(f"'{target_char}' not found in memory of {process_name}.")
```
请注意,这段代码仅适用于 Linux 系统,并且需要具有足够的权限来读取其他进程的内存。在 Windows 系统上,你可能需要使用其他方法来读取进程的内存。此外,读取其他进程的内存可能涉及安全风险,请谨慎使用。
用Python写一段程序,将txt文件中指定的文字全部删除。
可以使用Python中的文件操作和字符串处理函数来实现这个需求。以下是一个简单的示例代码:
```python
filename = "example.txt" # 文件名
target_word = "delete" # 要删除的目标词语
# 打开文件,读取内容
with open(filename, "r") as f:
content = f.read()
# 在内容中查找目标词语并删除
new_content = content.replace(target_word, "")
# 将修改后的内容写回文件
with open(filename, "w") as f:
f.write(new_content)
```
这段代码首先指定了要操作的文件名和要删除的目标词语。然后使用Python的文件操作打开文件并读取文件内容。接着使用字符串处理函数 `replace()` 在文件内容中查找并删除目标词语。最后将修改后的内容写回到文件中。
阅读全文
相关推荐
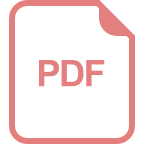
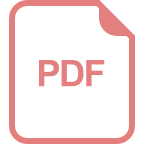
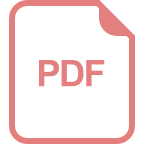
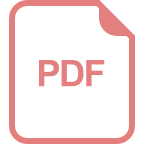
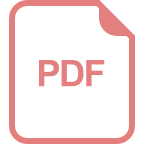
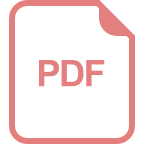
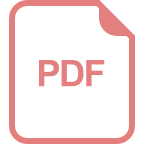
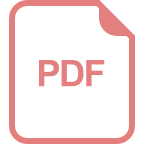
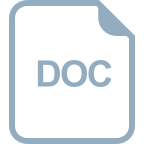




