Q = tf.keras.layers.Dense(queries, numUnits,activation='relu') # [64,10,300] TypeError: __init__() got multiple values for argument 'activation'
时间: 2023-12-19 11:07:18 浏览: 38
在使用 `tf.keras.layers.Dense()` 函数时,第一个参数应该是输出维度(即 `units`),第二个参数是输入维度(即 `input_shape` 或 `input_dim`),而不是输入张量。因此,您需要将 `queries` 作为 `input_shape` 或 `input_dim` 参数传递,而将 `numUnits` 作为 `units` 参数传递。
另外,`activation` 参数只能传递一个值,您可能在函数调用中重复传递了该参数。请检查您的代码并确保只有一个 `activation` 参数传递给 `tf.keras.layers.Dense()` 函数。
以下是使用正确参数调用 `tf.keras.layers.Dense()` 函数的示例代码:
```python
Q = tf.keras.layers.Dense(units=numUnits, activation='relu', input_shape=(queries,))
```
这应该能够解决您遇到的错误。
相关问题
def create_model(self): print('[NonLinearTransformer] Starting create_model') dense0 = tf.keras.layers.Dense(units=64, activation='relu') dense1 = tf.keras.layers.Dense(units=32, activation='relu') dense2 = tf.keras.layers.Dense(units=1, activation='linear') self.network = tf.keras.Sequential([ dense0, dense1, dense2], name='nonlineartransformer')
这段代码是用TensorFlow创建一个非线性变换模型的函数,具体实现如下:
```python
def create_model(self):
print('[NonLinearTransformer] Starting create_model')
dense0 = tf.keras.layers.Dense(units=64, activation='relu') # 创建一个具有64个神经元的全连接层,并使用ReLU激活函数
dense1 = tf.keras.layers.Dense(units=32, activation='relu') # 创建一个具有32个神经元的全连接层,并使用ReLU激活函数
dense2 = tf.keras.layers.Dense(units=1, activation='linear') # 创建一个具有1个神经元的输出层,并使用线性激活函数
self.network = tf.keras.Sequential([dense0, dense1, dense2], name='nonlineartransformer') # 将上面创建的三个层按顺序连接起来,构成一个完整的神经网络模型
```
该函数创建了一个包含三个全连接层的神经网络模型,其中前两个层使用ReLU激活函数,第三个层使用线性激活函数。该模型的输入和输出都是1维张量。
可以通过调用`model.summary()`方法来查看该模型的结构和参数数量。
Q = tf.layers.dense(queries, numUnits, activation=tf.nn.relu)在新版本的tensorflow中应该怎么写
在新版本的 TensorFlow 中,`tf.layers` 子模块已被弃用,其功能被转移到 `tf.keras.layers` 中。因此,您可以使用 `tf.keras.layers.Dense` 函数来替换 `tf.layers.dense`。
以下是使用 `tf.keras.layers.Dense` 函数的示例代码:
```python
Q = tf.keras.layers.Dense(units=numUnits, activation=tf.nn.relu)(queries)
```
这里,我们首先将 `numUnits` 作为 `units` 参数传递给 `tf.keras.layers.Dense` 函数,将 `tf.nn.relu` 作为 `activation` 参数传递给该函数,然后将 `queries` 作为输入张量传递给该函数。`tf.keras.layers.Dense` 的输出是一个张量,可以通过调用该函数并传递输入张量来获取它。
注意,如果您需要在 `tf.keras.layers.Dense` 函数之后添加其他层,请将每个层作为函数调用的一部分传递,就像上面的示例代码一样。
相关推荐
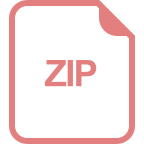
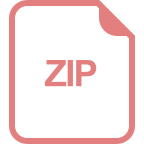
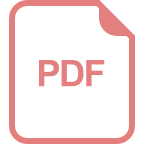













