编写一个C语言程序,读取一个数据文件(自定义一个大的整数文件),对每一个数据进行某种运算,再在屏幕输出计算结果。要求以上工作用3个进程实现,进程1负责读文件,进程2进行计算,进程3负责将计算结果输出到文件result.txt中。要求使用管道通信方式,请给出具体的代码
时间: 2024-03-23 13:38:05 浏览: 80
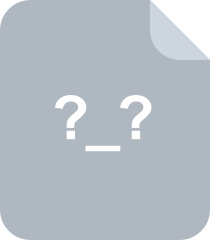
使用c语言读特定文件并在终端输出
以下是一个简单的示例代码,说明如何使用3个进程实现该需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/wait.h>
#define BUF_SIZE 256
int main() {
int fd[2];
pid_t pid[3];
// 创建管道
if (pipe(fd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
// 创建进程1,负责读取文件
pid[0] = fork();
if (pid[0] == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid[0] == 0) {
close(fd[0]); // 关闭读取端
int file_fd = open("data.txt", O_RDONLY);
if (file_fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
char buf[BUF_SIZE];
int n;
while ((n = read(file_fd, buf, BUF_SIZE)) > 0) {
if (write(fd[1], buf, n) != n) {
perror("write");
exit(EXIT_FAILURE);
}
}
close(file_fd);
close(fd[1]); // 关闭写入端
exit(EXIT_SUCCESS);
}
// 创建进程2,进行计算
pid[1] = fork();
if (pid[1] == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid[1] == 0) {
close(fd[1]); // 关闭写入端
int sum = 0;
char buf[BUF_SIZE];
int n;
while ((n = read(fd[0], buf, BUF_SIZE)) > 0) {
for (int i = 0; i < n; i++) {
// 对每一个数据进行某种运算
sum += buf[i];
}
}
printf("Sum: %d\n", sum);
close(fd[0]); // 关闭读取端
exit(EXIT_SUCCESS);
}
// 创建进程3,负责将计算结果输出到文件
pid[2] = fork();
if (pid[2] == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid[2] == 0) {
close(fd[0]); // 关闭读取端
int result_fd = open("result.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (result_fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
char buf[BUF_SIZE];
int n = sprintf(buf, "Sum: %d\n", sum);
if (write(result_fd, buf, n) != n) {
perror("write");
exit(EXIT_FAILURE);
}
close(result_fd);
close(fd[1]); // 关闭写入端
exit(EXIT_SUCCESS);
}
// 等待子进程结束
for (int i = 0; i < 3; i++) {
int status;
if (waitpid(pid[i], &status, 0) == -1) {
perror("waitpid");
exit(EXIT_FAILURE);
}
if (WIFEXITED(status)) {
printf("Child process %d exited with status %d\n", pid[i], WEXITSTATUS(status));
} else if (WIFSIGNALED(status)) {
printf("Child process %d terminated by signal %d\n", pid[i], WTERMSIG(status));
}
}
return 0;
}
```
该代码使用了3个进程,分别实现了读取文件、计算、输出结果三个任务,并通过管道进行通信。进程1读取数据文件,并通过管道将数据传递给进程2;进程2对每一个数据进行某种运算(这里简单地将每个数据的ASCII码值加起来),最终得到计算结果;进程3将计算结果输出到文件result.txt中。
阅读全文
相关推荐
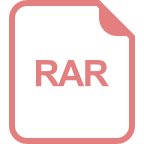


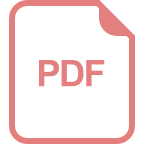
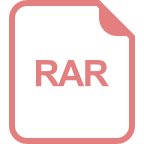
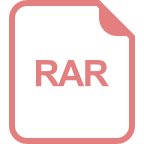
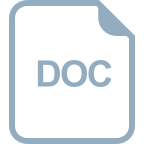
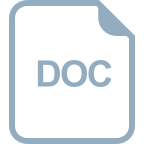
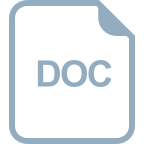
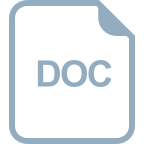
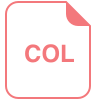
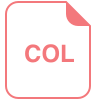
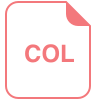
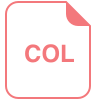
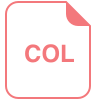
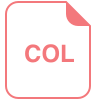