读取一个数据文件(自定义一个大的整数文件),对每一个数据进行某种运算,再在屏幕输出计算结果。要求以上工作用3个进程实现,进程1负责读文件,进程2进行计算,进程3负责将计算结果输出到文件result.txt中。要求使用管道通信方式.要求使用c语言进行编写
时间: 2024-03-24 08:36:31 浏览: 39
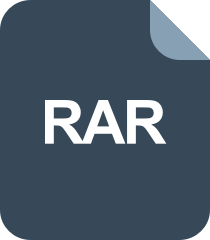
指定文件读取并输出出来
好的,以下是使用C语言编写的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#define DATA_FILE "data.txt"
#define RESULT_FILE "result.txt"
#define BUFFER_SIZE 1024
int main() {
int pipe1[2], pipe2[2], pipe3[2];
pid_t pid1, pid2, pid3;
// 创建管道
if (pipe(pipe1) < 0 || pipe(pipe2) < 0 || pipe(pipe3) < 0) {
perror("Error creating pipe");
exit(EXIT_FAILURE);
}
// 创建进程1
pid1 = fork();
if (pid1 < 0) {
perror("Error creating process 1");
exit(EXIT_FAILURE);
} else if (pid1 == 0) { // 进程1负责读文件
// 关闭管道2和管道3
close(pipe2[0]); close(pipe2[1]);
close(pipe3[0]); close(pipe3[1]);
// 打开数据文件
int fd = open(DATA_FILE, O_RDONLY);
if (fd < 0) {
perror("Error opening data file");
exit(EXIT_FAILURE);
}
char buffer[BUFFER_SIZE];
int size;
while ((size = read(fd, buffer, BUFFER_SIZE)) > 0) {
// 写入管道1
if (write(pipe1[1], buffer, size) < 0) {
perror("Error writing to pipe 1");
exit(EXIT_FAILURE);
}
}
// 关闭管道1
close(pipe1[0]); close(pipe1[1]);
close(fd);
exit(EXIT_SUCCESS);
}
// 创建进程2
pid2 = fork();
if (pid2 < 0) {
perror("Error creating process 2");
exit(EXIT_FAILURE);
} else if (pid2 == 0) { // 进程2负责计算
// 关闭管道1和管道3
close(pipe1[0]); close(pipe1[1]);
close(pipe3[0]); close(pipe3[1]);
// 从管道1读取数据
char buffer[BUFFER_SIZE];
int size = read(pipe2[0], buffer, BUFFER_SIZE);
if (size < 0) {
perror("Error reading from pipe 1");
exit(EXIT_FAILURE);
}
// 进行计算
char *ptr = buffer;
int sum = 0;
while (*ptr != '\0') {
char *end;
int num = strtol(ptr, &end, 10);
sum += num;
ptr = end;
}
// 将结果写入管道2
char result[BUFFER_SIZE];
sprintf(result, "%d", sum);
size = strlen(result);
if (write(pipe2[1], result, size) < 0) {
perror("Error writing to pipe 2");
exit(EXIT_FAILURE);
}
// 关闭管道2
close(pipe2[0]); close(pipe2[1]);
exit(EXIT_SUCCESS);
}
// 创建进程3
pid3 = fork();
if (pid3 < 0) {
perror("Error creating process 3");
exit(EXIT_FAILURE);
} else if (pid3 == 0) { // 进程3负责输出结果
// 关闭管道1和管道2
close(pipe1[0]); close(pipe1[1]);
close(pipe2[0]); close(pipe2[1]);
// 打开结果文件
int fd = open(RESULT_FILE, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd < 0) {
perror("Error opening result file");
exit(EXIT_FAILURE);
}
// 从管道3读取数据
char buffer[BUFFER_SIZE];
int size = read(pipe3[0], buffer, BUFFER_SIZE);
if (size < 0) {
perror("Error reading from pipe 3");
exit(EXIT_FAILURE);
}
// 将结果写入文件
if (write(fd, buffer, size) < 0) {
perror("Error writing to result file");
exit(EXIT_FAILURE);
}
// 关闭管道3和文件
close(pipe3[0]); close(pipe3[1]);
close(fd);
exit(EXIT_SUCCESS);
}
// 关闭父进程的管道
close(pipe1[0]); close(pipe1[1]);
close(pipe2[0]); close(pipe2[1]);
close(pipe3[0]); close(pipe3[1]);
// 等待子进程结束
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
waitpid(pid3, NULL, 0);
return 0;
}
```
以上代码中,我们首先定义了数据文件和结果文件路径,以及管道和缓冲区大小。然后创建了3个进程,分别负责读文件、进行计算和输出结果的功能。在每个进程中,我们分别关闭了不需要使用的管道,并实现了相应的功能。
需要注意的是,在进程1中,我们需要将文件数据写入管道1中;在进程2中,我们需要从管道1中读取数据并进行计算,然后将结果写入管道2中;在进程3中,我们需要从管道2中读取数据并将结果写入文件中。这样就完成了以上要求。
当然,以上代码只是一个简单的示例,实际情况中还需要考虑异常处理、数据清洗等问题。
阅读全文
相关推荐
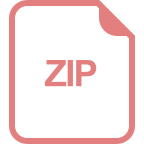
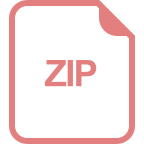

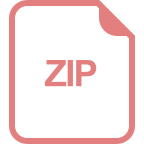
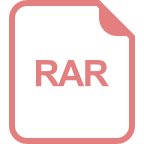
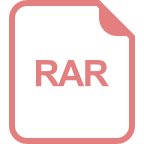
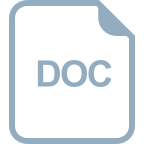
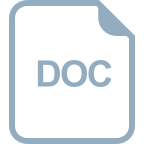
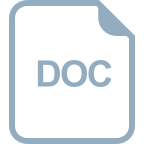
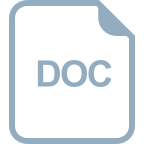
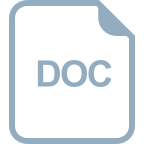
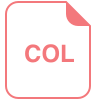
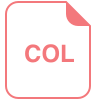
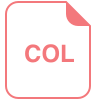
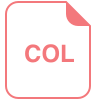