python爬虫爬取天气数据并以xlxs保存下来
时间: 2024-11-07 11:29:18 浏览: 55
在Python中爬取天气数据并保存到Excel文件(xls格式),通常需要几个步骤:
1. **选择天气API**:首先,你需要找到一个提供天气信息的API,比如中国气象局的API、OpenWeatherMap等。注册获取API密钥。
2. **安装库**:安装必要的库,如requests(用于发送HTTP请求)、BeautifulSoup(用于解析HTML)和pandas(处理数据和导出到Excel)。可以使用`pip install requests beautifulsoup4 pandas openpyxl`命令安装。
3. **编写代码**:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 使用API密钥和URL构建请求
api_url = "https://your_weather_api.com/weather"
headers = {
'Authorization': 'Bearer your_api_key',
'Content-Type': 'application/json'
}
def get_weather_data():
response = requests.get(api_url, headers=headers)
data = response.json()
# 提取所需的数据字段,例如城市名、日期和温度
city = data['city']
date = data['date']
temperature = data['temperature']
# 创建一个字典列表,存储每一天的天气信息
weather_list = [{'City': city, 'Date': date, 'Temperature': temperature}]
return weather_list
weather_data = get_weather_data()
# 将数据转换为DataFrame
df = pd.DataFrame(weather_data)
# 导出为Excel文件(xls格式)
df.to_excel('weather_data.xls', index=False)
```
**注意事项**:
- API的具体使用方式和返回的数据结构可能会因服务而异,请查阅文档调整相应部分。
- 对于某些API,可能还需要对JSON数据进行适当的处理才能提取所需字段。
- 如果API返回的是CSV或其他格式,可能需要额外的转换步骤。
阅读全文
相关推荐
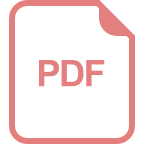
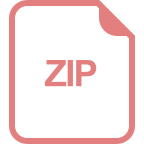
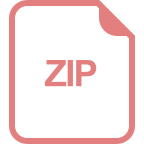















