SM4的CTR模式,使用C语言不借助库实现,代码示例
时间: 2024-09-08 13:00:56 浏览: 47
SM4(Stream Cipher Mode 4)是一种密码块加密标准,用于对数据流进行加密。CTR(Counter)模式是一种常用的密钥流生成方式,它通过将明文长度与密钥相结合,生成一个唯一的加密计数器(Counter),然后与密钥进行异或操作得到密钥流。
以下是使用C语言不借助外部库实现SM4 CTR模式的一个简单示例:
```c
#include <stdint.h>
// SM4 key (32 bytes) and initialization vector (IV, also 32 bytes)
uint8_t sm4_key[32], iv[32];
// SM4 S-boxes and other constants
const uint8_t sbox[256][4] = ...; // 实现SM4 S-box的具体数组
const uint32_t rcon[] = ...; // Rcon序列
// Function to perform AES-like SubBytes operation (SM4 S-box)
void sub_bytes(uint8_t* input) {
int i;
for (i = 0; i < 4; ++i) {
input[i] = sbox[input[i]];
}
}
// Function to perform the SM4 CTR encryption
uint8_t sm4_ctr_encrypt(const uint8_t* plaintext, size_t len) {
uint8_t keystream[16], counter[4];
size_t pos;
// Load IV into counter array
memcpy(counter, iv, sizeof(counter));
// XOR plaintext with keystream
for (pos = 0; pos < len; pos += 16) {
// Generate next keystream block
uint32_t keystream_word;
for (int i = 0; i < 4; ++i) {
keystream_word |= ((counter[i] << (8 * (3 - i))) ^ plaintext[pos + i]) & 0xFF;
}
// Apply SubBytes and MixColumns transformations
sub_bytes((uint8_t*)&keystream_word);
for (int j = 0; j < 4; ++j) {
keystream_word = mix_columns(keystream_word, j);
}
// Update counter
counter[3]++;
if (counter[3] == 0) {
counter[2]++; // Roll over after reaching FF
if (counter[2] == 0) {
counter[1]++;
if (counter[1] == 0) {
counter[0]++;
if (counter[0] == 0 && rcon[0]) { // Use first Rcon on IV if needed
sub_bytes(counter);
for (int i = 0; i < 4; ++i) {
keystream_word ^= rcon[0] << (8 * i);
}
}
}
}
}
// XOR plaintext with keystream
for (int i = 0; i < 16; ++i) {
plaintext[pos + i] ^= keystream[i];
}
}
return plaintext;
}
```
注意:此代码仅作教学演示,实际应用中需要确保所有S-box、MixColumns和其他密钥流生成细节都准确无误地实现了SM4规范。由于SM4相对复杂,这里省略了许多细节,如轮函数的实现、循环移位等。此外,真正的实现通常会考虑性能优化和安全性考虑,比如内联S-box运算、缓存友好的内存访问等。
相关推荐
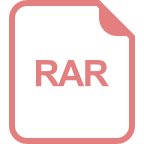
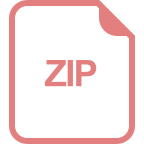














