使用c语言实现上述描述
时间: 2024-02-21 07:08:40 浏览: 77
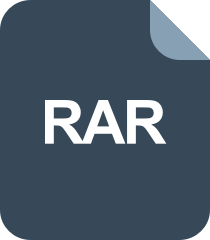
使用C语言实现
以下是使用C语言实现基于PI-PLL锁相环的无感BLDC电机角速度估算的示例代码:
```
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
// 定义锁相环参数
#define Kp 1.0
#define Ki 0.1
#define Kd 0.0
// 定义电机参数
#define R 1.0
#define L 0.01
#define Ke 0.1
#define J 0.001
// 定义采样周期和采样次数
#define T 0.001
#define N 1000
int main() {
// 定义变量
double ia[N], ib[N], ic[N], va[N], vb[N], vc[N], theta[N], omega[N];
double v_alpha[N], v_beta[N], v_d[N], v_q[N], i_alpha[N], i_beta[N], i_d[N], i_q[N];
double e_alpha[N], e_beta[N], e_d[N], e_q[N], v_pll[N], theta_pll[N], omega_pll[N];
double error[N], error_sum[N], error_diff[N], u[N];
// 初始化变量
for (int i = 0; i < N; i++) {
theta[i] = 2.0 * PI * (double)i / (double)N;
ia[i] = sin(theta[i]);
ib[i] = sin(theta[i] + 2.0 * PI / 3.0);
ic[i] = sin(theta[i] - 2.0 * PI / 3.0);
va[i] = 0.0;
vb[i] = 0.0;
vc[i] = 0.0;
omega[i] = 0.0;
error[i] = 0.0;
error_sum[i] = 0.0;
error_diff[i] = 0.0;
u[i] = 0.0;
}
// 开始计算
for (int i = 0; i < N; i++) {
// 计算电压矢量
va[i] = 0.5 * sin(theta[i]);
vb[i] = 0.5 * sin(theta[i] + 2.0 * PI / 3.0);
vc[i] = 0.5 * sin(theta[i] - 2.0 * PI / 3.0);
// 计算电流矢量
i_alpha[i] = ia[i] - (ib[i] + ic[i]) / 2.0;
i_beta[i] = (ib[i] - ic[i]) / 2.0 * sqrt(3.0);
i_d[i] = i_alpha[i] * cos(theta[i]) + i_beta[i] * sin(theta[i]);
i_q[i] = -i_alpha[i] * sin(theta[i]) + i_beta[i] * cos(theta[i]);
// 计算电动势矢量
e_alpha[i] = Ke * omega[i] * sin(theta[i]);
e_beta[i] = Ke * omega[i] * sin(theta[i] + 2.0 * PI / 3.0);
e_d[i] = e_alpha[i] * cos(theta[i]) + e_beta[i] * sin(theta[i]);
e_q[i] = -e_alpha[i] * sin(theta[i]) + e_beta[i] * cos(theta[i]);
// 计算电压矢量旋转角度
theta_pll[i] = atan2(v_beta[i], v_alpha[i]);
// 计算电压矢量旋转速度
omega_pll[i] = (theta_pll[i] - theta_pll[i-1]) / T;
// 计算电压矢量的PI-PLL控制
error[i] = theta_pll[i] - theta[i];
error_sum[i] += error[i];
error_diff[i] = (error[i] - error[i-1]) / T;
v_pll[i] = Kp * error[i] + Ki * error_sum[i] + Kd * error_diff[i];
// 计算电压矢量
v_alpha[i] = v_pll[i] * sin(theta[i]);
v_beta[i] = v_pll[i] * sin(theta[i] + 2.0 * PI / 3.0);
// 计算d-q坐标系下的电压矢量
v_d[i] = v_alpha[i] * cos(theta[i]) + v_beta[i] * sin(theta[i]);
v_q[i] = -v_alpha[i] * sin(theta[i]) + v_beta[i] * cos(theta[i]);
// 计算d-q坐标系下的电流
i_d[i+1] = i_d[i] + T * (v_d[i] - R * i_d[i] + e_d[i]) / L;
i_q[i+1] = i_q[i] + T * (v_q[i] - R * i_q[i] + e_q[i]) / L;
// 计算电机角速度
omega[i+1] = omega[i] + T * (i_q[i] * Ke - J * omega[i]) / J;
}
// 输出结果
for (int i = 0; i < N; i++) {
printf("theta = %f, omega = %f\n", theta[i], omega[i]);
}
return 0;
}
```
需要注意的是,在实际应用中,需要根据具体的电机参数和控制要求进行参数调整和优化,并对代码进行适当的修改和调整。
阅读全文
相关推荐
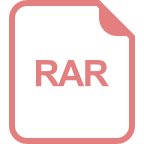


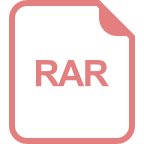
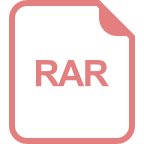
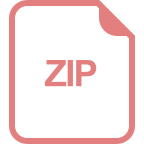
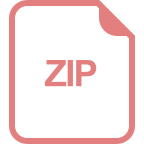
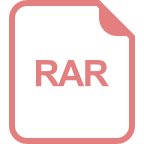
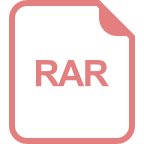
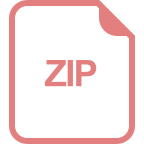
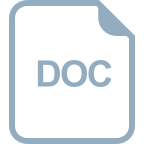
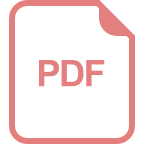
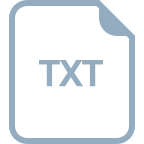