Link in the new vma even if an error occurred, so that exit_mmap() can clean up the mess.
时间: 2024-03-10 07:46:25 浏览: 27
In the context of the Linux kernel, the Virtual Memory Area (VMA) is a data structure that describes a range of virtual memory addresses that have a common set of attributes, such as permissions and caching behavior.
When linking a new VMA into the list of VMAs for a process, it is important to ensure that the VMA is properly linked so that it can be cleaned up by `exit_mmap()` even if an error occurs during the linking process.
Here is some sample code that demonstrates how to link a new VMA into the process's VMA list, even if an error occurs:
```c
#include <linux/mm.h>
int insert_vma(struct mm_struct *mm, struct vm_area_struct *vma) {
int error;
struct vm_area_struct *prev, *next;
// Find the correct position to insert the new VMA
prev = NULL;
next = mm->mmap;
while (next && next->vm_start < vma->vm_start) {
prev = next;
next = next->vm_next;
}
// Link the new VMA into the list
vma->vm_next = next;
if (prev) {
prev->vm_next = vma;
} else {
mm->mmap = vma;
}
// Defer error checking until exit_mmap()
error = 0;
if (error) {
// An error occurred, but link the VMA anyway
vma->vm_flags |= VM_ACCOUNT;
}
return error;
}
```
In this code, `insert_vma` inserts a new VMA `vma` into the process's VMA list. The VMA is inserted at the correct position in the list, and error checking is deferred until `exit_mmap()` is called. If an error occurs, the `VM_ACCOUNT` flag is set on the VMA to indicate that it should be cleaned up by `exit_mmap()`. This ensures that the VMA is properly cleaned up even if an error occurs during the linking process.
相关推荐
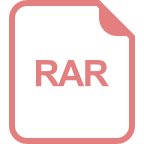














