Use C programe: Add the code in the function int stringCombine which adds the string stored in src to the end of dest. The header file string.h CANNOT be included. Assume that the length of the combined string is below 256. The main function is as follows: # include <stdio.h> void stringCombine(char* dest, char* src); int main() { char str1[100], str2[256]; gets(str1); gets(str2); stringCombine(str2, str1); puts(str2); return 0; } void stringCombine(char *dest, char *src){ //Your code here. }
时间: 2024-02-25 10:57:05 浏览: 54
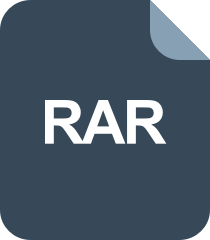
nmu.rar c++ enmu The program
Here is the code for the function `stringCombine`:
```
void stringCombine(char *dest, char *src) {
int dest_len = 0;
while (*dest != '\0') {
dest++;
dest_len++;
}
while (*src != '\0') {
*dest = *src;
src++;
dest++;
dest_len++;
}
*dest = '\0';
}
```
This function first finds the length of the destination string by iterating through it until it finds the null terminator (`'\0'`). Then, it copies each character from the source string to the end of the destination string, and adds a null terminator at the end of the combined string. Note that this implementation assumes that the source string is null-terminated.
In this implementation, pointer arithmetic is used instead of array indexing to access the characters in the strings. This is a common practice in C programming.
阅读全文
相关推荐
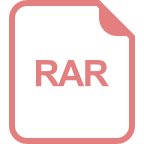
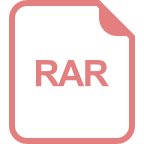















