C++封装stack
时间: 2024-10-11 19:13:24 浏览: 22
在C++中,Stack(栈)是一种线性的数据结构,遵循"先进后出"(Last In First Out, LIFO)的原则。它通常通过容器库中的`std::stack`模板类来实现,该模板允许你使用泛型,支持任意类型的元素。
`std::stack`包含的基本操作包括:
1. **push**:在栈顶添加一个新元素。
2. **pop**:从栈顶移除并返回一个元素,如果栈为空则抛出异常。
3. **top**:查看但不移除栈顶元素,获取其引用或值。
4. **empty**:检查栈是否为空。
5. **size**:获取当前栈中的元素个数。
下面是一个简单的例子展示了如何使用`std::stack`:
```cpp
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack; // 创建一个空的整数栈
// 入栈
myStack.push(10);
myStack.push(20);
myStack.push(30);
// 查看栈顶元素但不移除
int topElement = myStack.top(); // 此时topElement的值为30
// 出栈
myStack.pop();
std::cout << "Top element after pop: " << myStack.top() << "\n";
// 检查栈是否为空
if (myStack.empty()) {
std::cout << "Stack is empty.\n";
} else {
std::cout << "Stack size: " << myStack.size() << "\n"; // 输出3,因为还有两个元素
}
return 0;
}
```
阅读全文
相关推荐
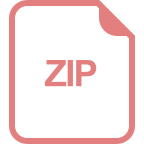
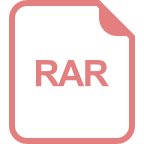
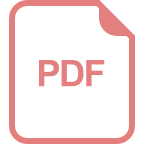
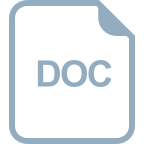

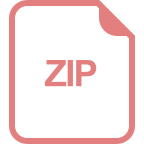
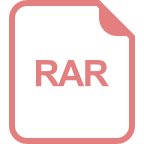
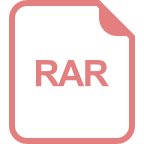
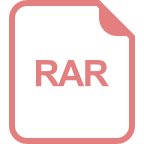
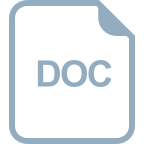
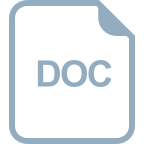
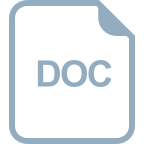
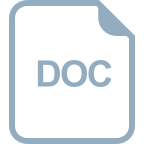
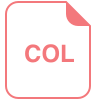
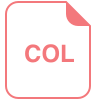
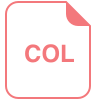
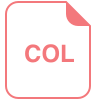

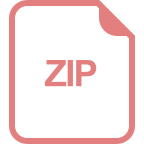