Intrinsic interpretable GNNs编程代码
时间: 2024-12-06 16:22:28 浏览: 9
Intrinsic interpretable Graph Neural Networks (IGNN)是一种专注于提高图神经网络内在可解释性的模型。这类模型通常会设计特殊的架构或者集成解释性强的操作,如节点特征选择、注意力机制等,使得生成的预测结果更容易理解。
编程代码示例(使用PyTorch Geometric库)可能会涉及以下几个步骤:
```python
import torch
import torch_geometric.nn as gnn
class IntrinsicIGNN(torch.nn.Module):
def __init__(self, in_channels, hidden_channels, num_layers, dropout=0.5):
super(IntrinsicIGNN, self).__init__()
# 初始化层,包括一个基础的图卷积层作为开始
self.conv_list = []
for _ in range(num_layers):
if _ == 0:
self.conv_list.append(gnn.GCNConv(in_channels, hidden_channels))
else:
self.conv_list.append(gnn.GraphConv(hidden_channels, hidden_channels)) # 后续的层通常保持相同的隐藏通道数
self.conv_list.append(gnn.Dropout(dropout))
# 添加可解释性操作,比如注意力模块
self.interpretable_layer = AttentionModule(hidden_channels)
def forward(self, x, edge_index):
h = x
for conv in self.conv_list[:-1]:
h = conv(h, edge_index)
h = F.relu(h)
h = F.dropout(h, training=self.training)
# 应用可解释性层
attended_h = self.interpretable_layer(h, edge_index)
return attended_h # 返回经过解释性处理后的节点表示
# 自定义注意力模块
class AttentionModule(torch.nn.Module):
def __init__(self, hidden_channels):
super(AttentionModule, self).__init__()
self.attention_weights = torch.nn.Parameter(torch.Tensor(hidden_channels)) # 存储注意力权重
def forward(self, features, edge_index):
alpha = torch.softmax(torch.matmul(features, self.attention_weights), dim=-1) # 计算注意力分数
aggregated = torch.spmm(alpha, features) # 根据注意力得分加权求和
return aggregated
```
阅读全文
相关推荐
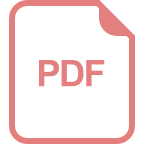
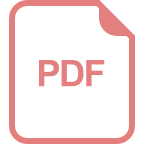
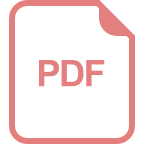















