for in python
时间: 2023-08-14 16:14:27 浏览: 84
In Python, the `for` loop is used to iterate over a sequence (such as a list, tuple, or string) or other iterable objects. It allows you to perform a set of statements repeatedly for each item in the sequence.
The basic syntax of a `for` loop in Python is as follows:
```python
for item in sequence:
# code to be executed for each item
```
Here, `item` represents the current item in the sequence, and `sequence` is the iterable object. Within the loop, you can perform operations using the `item` variable.
For example, let's say you have a list of numbers and you want to print each number using a `for` loop:
```python
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
```
This will output:
```
1
2
3
4
5
```
You can also use the `range()` function to generate a sequence of numbers and iterate over it:
```python
for i in range(1, 6):
print(i)
```
This will produce the same output as the previous example.
In addition, you can use the `enumerate()` function to iterate over a sequence while also getting the index of each item. Here's an example:
```python
fruits = ["apple", "banana", "orange"]
for index, fruit in enumerate(fruits):
print(index, fruit)
```
This will output:
```
0 apple
1 banana
2 orange
```
I hope this helps! Let me know if you have any more questions.
阅读全文
相关推荐
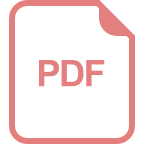
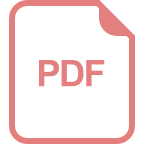
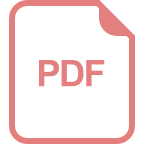
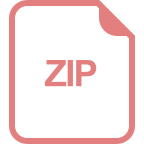
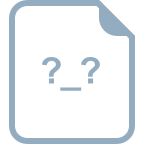
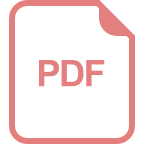
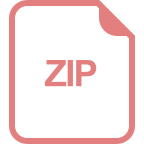
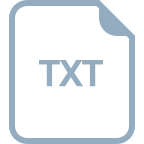
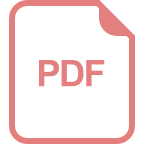
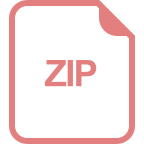
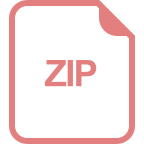
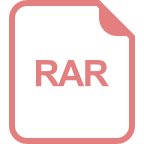
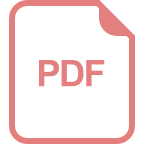
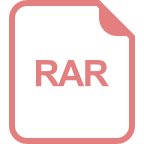
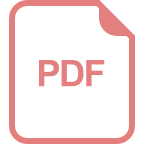