用c++整理顺序表的基本运算(InitList、StoreList、ReadList、GetNode、LocateNode) 要求:保存5个数,数据自定
时间: 2024-09-10 15:28:28 浏览: 54
在C++中,我们通常使用数组或者动态内存分配的结构体(如`std::vector`)来表示顺序表。这里是一个简单的例子,展示了如何使用C++实现基本的顺序表操作,比如初始化列表(InitList)、存储元素(StoreList)、读取元素(ReadList)、获取节点值(GetNode)以及查找节点位置(LocateNode)。
```cpp
#include <iostream>
using namespace std;
// 定义顺序表节点结构
struct ListNode {
int data;
ListNode* next;
};
// 初始化列表函数
void InitList(ListNode** head, const int count, int value = 0) {
*head = new ListNode();
(*head)->data = value;
(*head)->next = nullptr;
for (int i = 1; i < count; ++i) {
ListNode* newNode = new ListNode(value);
newNode->next = *head;
*head = newNode;
}
}
// 存储元素函数
void StoreList(ListNode** head, int index, int value) {
if (index >= 0 && index < GetListNodeCount(*head)) {
(*head)->next[index].data = value;
} else {
cout << "Index out of range!" << endl;
}
}
// 读取元素函数
int ReadList(const ListNode* head, int index) {
if (index >= 0 && index < GetListNodeCount(head)) {
return head->next[index].data;
} else {
cout << "Index out of range!" << endl;
return -1;
}
}
// 获取节点值函数
int GetNode(const ListNode* head, int index) {
return head->next[index].data;
}
// 查找节点位置函数
int LocateNode(const ListNode* head, int value) {
for (int i = 0, pos = 0; head->next != nullptr; ++pos, head = head->next) {
if (head->data == value) {
return pos;
}
}
// 如果未找到,返回-1
return -1;
}
// 计算顺序表长度的辅助函数
int GetListNodeCount(const ListNode* head) {
int count = 0;
while (head->next != nullptr) {
++count;
head = head->next;
}
return count;
}
// 示例用法
int main() {
ListNode* listHead = nullptr;
InitList(&listHead, 5, 1); // 初始化一个包含5个1的顺序表
// 存取元素
StoreList(&listHead, 0, 2);
cout << "Element at index 0 is: " << ReadList(listHead, 0) << endl;
// 查找元素位置
int position = LocateNode(listHead, 2);
if (position != -1) {
cout << "Found element 2 at index: " << position << endl;
}
return 0;
}
```
阅读全文
相关推荐
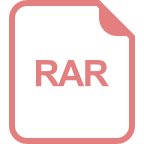
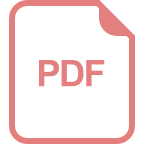
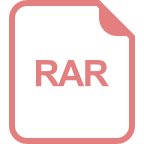
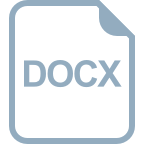
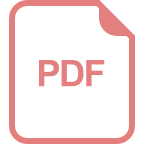
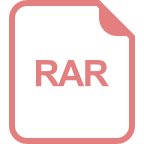
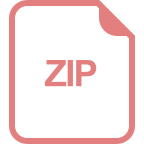
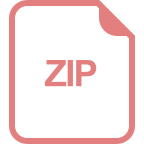
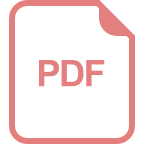
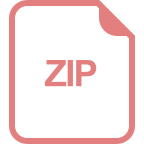
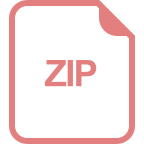
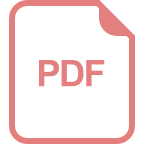
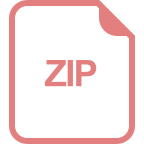
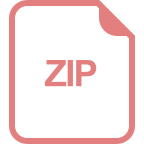