c语言成绩管理系统文件保存方式
时间: 2024-12-16 18:21:07 浏览: 11
在C语言中创建一个成绩管理系统,通常会涉及文件操作,以便持久化存储学生的成绩信息。一种常见的保存方式是使用文本文件,比如`.txt`格式,因为C语言的标准库`stdio.h`提供了读写文本文件的功能。
首先,你可以选择将数据结构(如学生姓名、学号和成绩的结构体)转换为字符串并写入文件,例如:
```c
typedef struct {
char name[50];
int id;
float score;
} Student;
void save_scores_to_file(Student* students, int count, const char* filename) {
FILE* file = fopen(filename, "w");
if (file != NULL) {
for (int i = 0; i < count; i++) {
fprintf(file, "%s %d %.2f\n", students[i].name, students[i].id, students[i].score);
}
fclose(file);
} else {
printf("Error opening the file.\n");
}
}
```
这里我们打开文件(如果成功),遍历学生数组,将每个学生的数据写入文件,每行包含姓名、学号和成绩,并在记录之间添加换行符`\n`。
另一种方式是使用二进制文件(`.bin`),适合存储原始数值,性能更高,但处理起来相对复杂些:
```c
void save_scores_to_binary(Student* students, int count, const char* filename) {
FILE* file = fopen(filename, "wb");
if (file != NULL) {
for (int i = 0; i < count * sizeof(Student); i += sizeof(Student)) {
fwrite(&students[i / sizeof(Student)], 1, sizeof(Student), file);
}
fclose(file);
} else {
printf("Error opening the file.\n");
}
}
```
在这段代码里,我们使用`fwrite`函数以字节为单位写入学生数据到二进制文件。
阅读全文
相关推荐





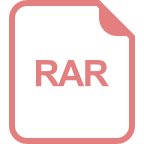











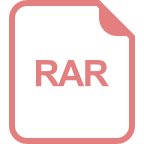
