c++,读取csv文件,每一行有4个数据,前两个数据是一对(X,Y),后两个数据是一对(X,Y)。读取整个csv文件,生成2个数组。
时间: 2024-12-17 15:36:27 浏览: 11
在C++中,你可以使用标准库如`fstream`来读取CSV文件,并利用`std::getline`函数逐行读取数据。对于每行,你可以使用`std::stringstream`来分割数据,创建两个包含(X, Y)对的数组。这里是一个简单的示例:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <string>
// 定义一个结构体用于存储一对(X, Y)
struct Coordinate {
double x, y;
};
// 从CSV字符串解析出Coordinate对
void parseXY(const std::string& csvLine, std::vector<Coordinate>& coordinates) {
std::istringstream iss(csvLine);
std::string item;
Coordinate coord;
// 遍历每一项,获取X和Y
while (iss >> item) {
if (item.find(',') != std::string::npos) {
double valX = std::stod(item.substr(0, item.find(',')));
double valY = std::stod(item.substr(item.find(',') + 1));
coord.x = valX;
coord.y = valY;
coordinates.push_back(coord); // 添加到坐标数组
} else {
break; // 如果不是一个完整的(X, Y),跳出循环
}
}
}
int main() {
std::ifstream inputFile("your_file.csv"); // 替换为你的CSV文件路径
if (!inputFile.is_open()) {
std::cerr << "Failed to open file!" << std::endl;
return 1;
}
std::vector<Coordinate> xy1, xy2; // 两个分别存储(X, Y)对的数组
std::string line;
while (std::getline(inputFile, line)) { // 逐行读取
if (!line.empty()) { // 排除空行
parseXY(line, xy1); // 解析X和Y到xy1
// 如果需要,可以为第二个数组xy2分配同样的逻辑
}
}
inputFile.close();
// 使用xy1和xy2数组
// ...
return 0;
}
```
阅读全文
相关推荐



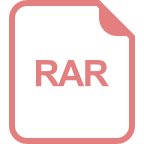














