给定一个非负的递增序列,实现完全二叉搜索树的建立,C语言
时间: 2024-12-13 11:19:51 浏览: 24
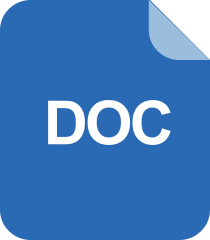
二叉排序树的建立、插入、删除和查找.doc
在C语言中,我们可以使用迭代的方式来构建一个完全二叉搜索树,给定一个非负递增的整数序列。完全二叉搜索树的特性是每个节点的值大于左子树中所有节点的值,小于右子树中所有节点的值。下面是使用数组表示的简单步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体代表二叉树节点
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建一个新的节点
TreeNode* newNode(int val) {
TreeNode* node = (TreeNode*)malloc(sizeof(TreeNode));
if (node) {
node->val = val;
node->left = NULL;
node->right = NULL;
}
return node;
}
// 插入函数,用于构建二叉搜索树
TreeNode* insertIntoBST(TreeNode* root, int val) {
if (!root) {
return newNode(val);
}
if (val < root->val) {
root->left = insertIntoBST(root->left, val);
} else if (val > root->val) {
root->right = insertIntoBST(root->right, val);
}
return root;
}
// 使用给定的递增序列构造完全二叉搜索树
void buildBSTFromSortedArray(int arr[], int n, TreeNode** root) {
*root = NULL;
for (int i = 0; i < n; ++i) {
(*root) = insertIntoBST(*root, arr[i]);
}
}
// 打印二叉搜索树
void inorderTraversal(TreeNode* root) {
if (root) {
inorderTraversal(root->left);
printf("%d ", root->val); // 左 -> 根 -> 右
inorderTraversal(root->right);
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
TreeNode* root = NULL;
buildBSTFromSortedArray(arr, n, &root);
printf("Root of the BST: ");
inorderTraversal(root);
return 0;
}
```
在这个程序中,`buildBSTFromSortedArray` 函数接受一个有序数组和它的长度,然后逐个元素插入到二叉搜索树中。最后通过 `inorderTraversal` 函数展示出构建后的完整二叉搜索树。
阅读全文
相关推荐
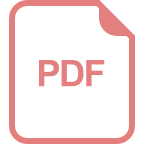
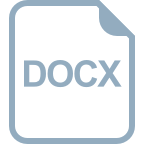
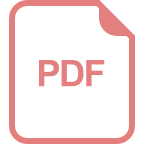
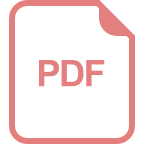
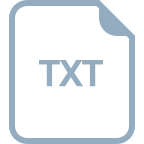
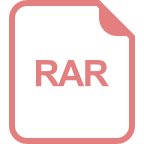
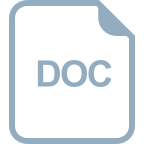
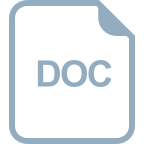



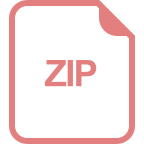