数据结构c++顺序栈
时间: 2023-11-30 20:38:19 浏览: 92
好的,关于数据结构中的顺序栈,它是一种基于数组实现的栈结构,具有后进先出(LIFO)的特点。下面是一个简单的C++实现:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 栈的最大容量
class SeqStack {
private:
int data[MAXSIZE]; // 存储栈中元素的数组
int top; // 栈顶指针,指向栈顶元素的下一个位置
public:
SeqStack() { top = 0; } // 构造函数,初始化栈顶指针为0
bool isEmpty() { return top == 0; } // 判断栈是否为空
bool isFull() { return top == MAXSIZE; } // 判断栈是否已满
void push(int x) { // 元素x入栈
if (isFull()) {
cout << "Stack overflow!" << endl;
return;
}
data[top++] = x;
}
void pop() { // 栈顶元素出栈
if (isEmpty()) {
cout << "Stack underflow!" << endl;
return;
}
top--;
}
int getTop() { // 获取栈顶元素
if (isEmpty()) {
cout << "Stack is empty!" << endl;
return -1;
}
return data[top-1];
}
};
int main() {
SeqStack s;
s.push(1);
s.push(2);
s.push(3);
cout << s.getTop() << endl; // 输出3
s.pop();
cout << s.getTop() << endl; // 输出2
s.pop();
cout << s.getTop() << endl; // 输出1
s.pop();
s.pop(); // 输出"Stack underflow!"
return 0;
}
```
阅读全文
相关推荐
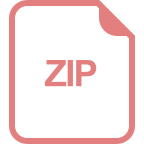
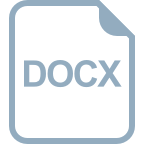
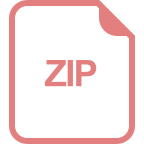
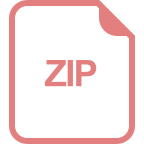
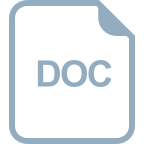
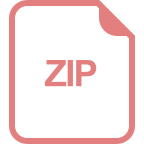
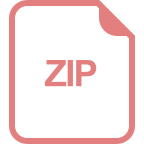
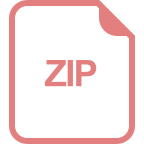
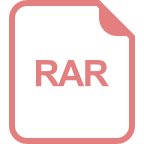
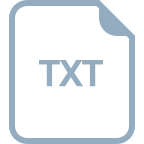
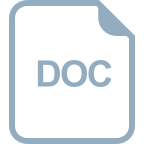
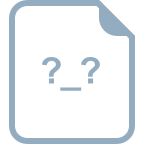
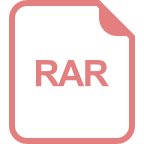