用A*算法写一段寻找多个目标的寻路算法代码
时间: 2024-05-08 21:22:31 浏览: 197
以下是用A*算法寻找多个目标的寻路算法代码:
```python
from queue import PriorityQueue
def a_star(start, targets, graph):
open_set = PriorityQueue()
open_set.put((0, start))
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic(start, targets)}
while not open_set.empty():
current = open_set.get()[1]
if current in targets:
return reconstruct_path(came_from, current)
for neighbor in graph[current]:
tentative_g_score = g_score[current] + distance(current, neighbor)
if neighbor not in g_score or tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = tentative_g_score + heuristic(neighbor, targets)
open_set.put((f_score[neighbor], neighbor))
return None
def heuristic(node, targets):
return min(distance(node, target) for target in targets)
def distance(node1, node2):
# assume Euclidean distance
return ((node1[0] - node2[0]) ** 2 + (node1[1] - node2[1]) ** 2) ** 0.5
def reconstruct_path(came_from, current):
path = [current]
while current in came_from:
current = came_from[current]
path.append(current)
path.reverse()
return path
```
这个算法的输入参数包括起始位置`start`,目标位置的列表`targets`,以及有向图`graph`,其中`graph`是一个字典,键为节点,值为该节点能够到达的所有邻居节点。输出是从起始位置到某个目标位置的最短路径。算法中用到了优先队列来存储待选节点,每次取出f值最小的节点进行扩展。`heuristic`函数用于估计当前节点到目标节点的距离,`distance`函数用于计算两个节点之间的距离,`reconstruct_path`函数用于重构最短路径。
阅读全文
相关推荐
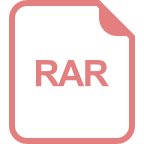
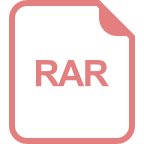
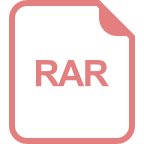
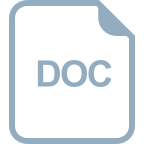
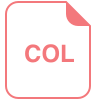
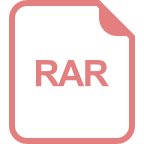
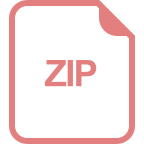
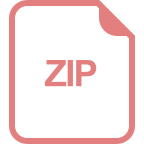
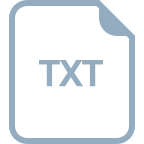
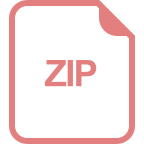
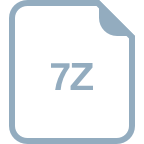
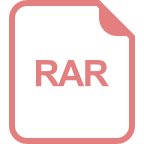
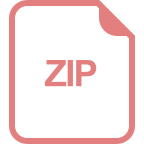
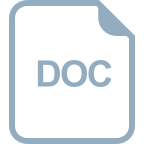
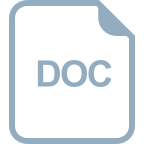
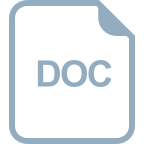
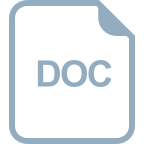