JAVA代码连接数据库,数据库内两个表,一个读者表(要求有账号和密码两个数据)进行增查功能,一个图书表(要求有书名,ISBN,作者,出版社四个数据)进行增删改查功能。
时间: 2024-03-08 19:46:28 浏览: 15
以下是基于 JDBC 的 Java 代码连接数据库,并实现读者表和图书表的增删改查功能的示例:
```java
import java.sql.*;
public class DatabaseConnection {
// 数据库连接信息
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost:3306/library";
static final String USER = "root";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
// 注册 JDBC 驱动
Class.forName(JDBC_DRIVER);
// 打开连接
System.out.println("连接数据库...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
// 执行查询
System.out.println("创建读者表...");
stmt = conn.createStatement();
String sql = "CREATE TABLE readers " +
"(id INTEGER not NULL, " +
" account VARCHAR(255), " +
" password VARCHAR(255), " +
" PRIMARY KEY ( id ))";
stmt.executeUpdate(sql);
System.out.println("创建图书表...");
sql = "CREATE TABLE books " +
"(id INTEGER not NULL, " +
" name VARCHAR(255), " +
" isbn VARCHAR(255), " +
" author VARCHAR(255), " +
" publisher VARCHAR(255), " +
" PRIMARY KEY ( id ))";
stmt.executeUpdate(sql);
// 执行增加操作
System.out.println("插入读者数据...");
sql = "INSERT INTO readers (id, account, password) VALUES (1, 'test', '123456')";
stmt.executeUpdate(sql);
System.out.println("插入图书数据...");
sql = "INSERT INTO books (id, name, isbn, author, publisher) VALUES (1, 'Java编程思想', '978-7-121-15695-2', 'Bruce Eckel', '机械工业出版社')";
stmt.executeUpdate(sql);
// 执行查询操作
System.out.println("查询读者数据...");
sql = "SELECT * FROM readers";
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String account = rs.getString("account");
String password = rs.getString("password");
System.out.print("ID: " + id);
System.out.print(", 账号: " + account);
System.out.println(", 密码: " + password);
}
System.out.println("查询图书数据...");
sql = "SELECT * FROM books";
rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String isbn = rs.getString("isbn");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
System.out.print("ID: " + id);
System.out.print(", 书名: " + name);
System.out.print(", ISBN: " + isbn);
System.out.print(", 作者: " + author);
System.out.println(", 出版社: " + publisher);
}
// 执行更新操作
System.out.println("更新图书数据...");
sql = "UPDATE books SET name='Java核心技术', author='Cay S. Horstmann' WHERE id=1";
int rows = stmt.executeUpdate(sql);
System.out.println(rows + " 行数据被更新!");
// 执行删除操作
System.out.println("删除图书数据...");
sql = "DELETE FROM books WHERE id=1";
rows = stmt.executeUpdate(sql);
System.out.println(rows + " 行数据被删除!");
// 关闭资源
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
// 处理 JDBC 错误
se.printStackTrace();
} catch (Exception e) {
// 处理 Class.forName 错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
该示例代码中使用的是 MySQL 数据库,需要提前在本地安装并配置好。同时,还需要在 MySQL 中创建名为 "library" 的数据库。
在代码中,首先注册 JDBC 驱动,然后打开数据库连接,创建读者表和图书表,插入数据,查询数据,更新数据,删除数据。最后关闭资源。
以上就是一个简单的 Java 代码连接数据库,并实现增删改查功能的示例。
相关推荐
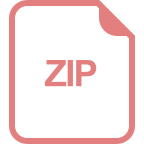
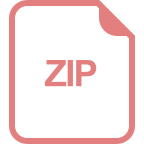
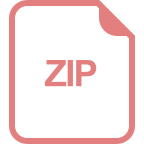














